1.SpringBoot概述
- Spring Boot是Spring提供的一个子项目,用于快速构建Spring应用程序
1.1 SpringBoot特性
1.1.1 起步依赖
1 | 本质上就是一个Maven坐标,整合了完成一个功能需要的所有坐标 |
1.1.2 自动配置
1 | 遵循约定大约配置的原则,在boot程序启动后,一些bean对象会自动注入到ioc容器,不需要手动声明,简化开发 |
1.1.3 其它特性
1 | 1.内嵌的Tomcat、Jetty(无需部署WAR文件,只需要jar包即可) |
2. SpringBoot快速配置启动
- 需求:
- 使用步骤:
2.1 创建springboot项目(需要联网)
基于Spring官方骨架,创建SpringBoot工程
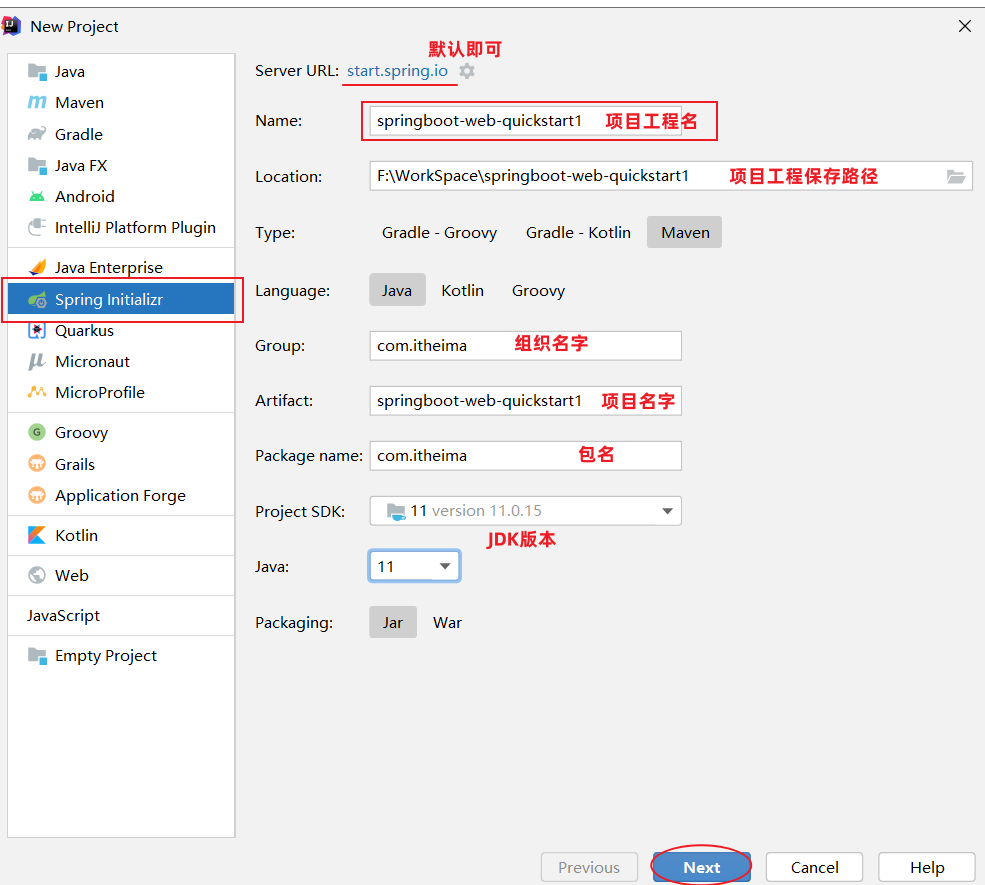
基本信息描述完毕之后,勾选web开发相关依赖
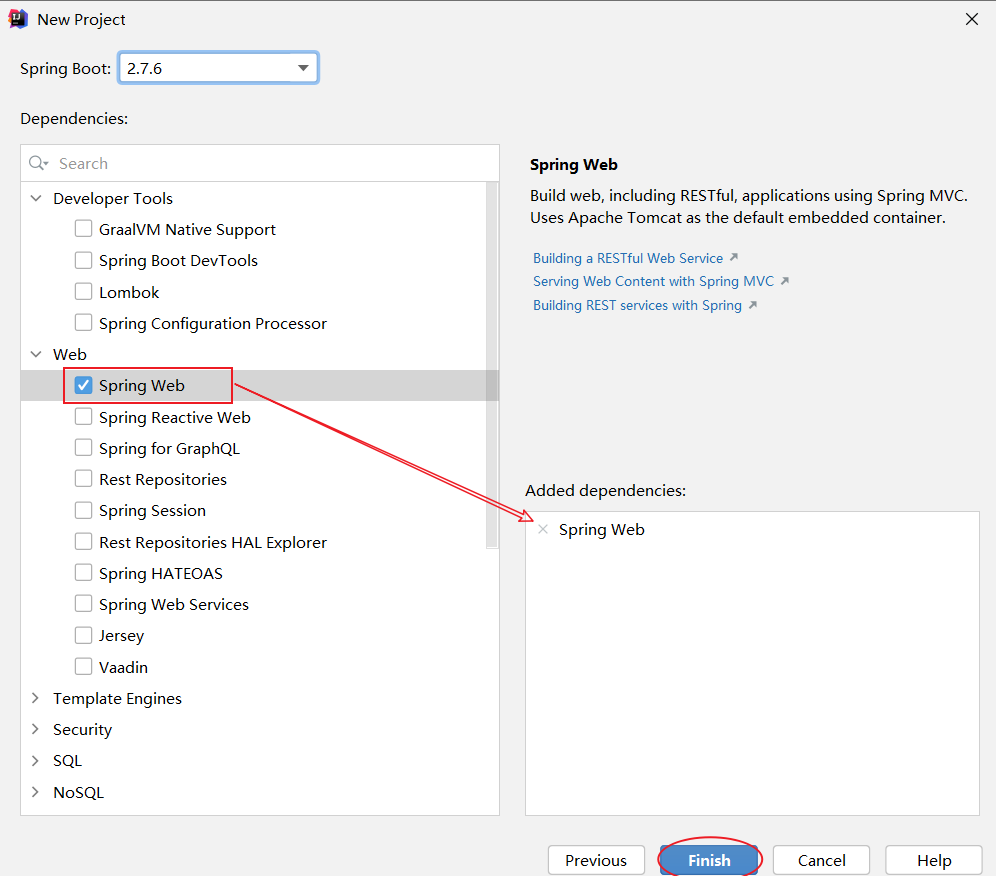
点击Finish之后,就会联网创建这个SpringBoot工程,创建好之后,结构如下:
2.2 定义请求处理类
运行SpringBoot自动生成的引导类
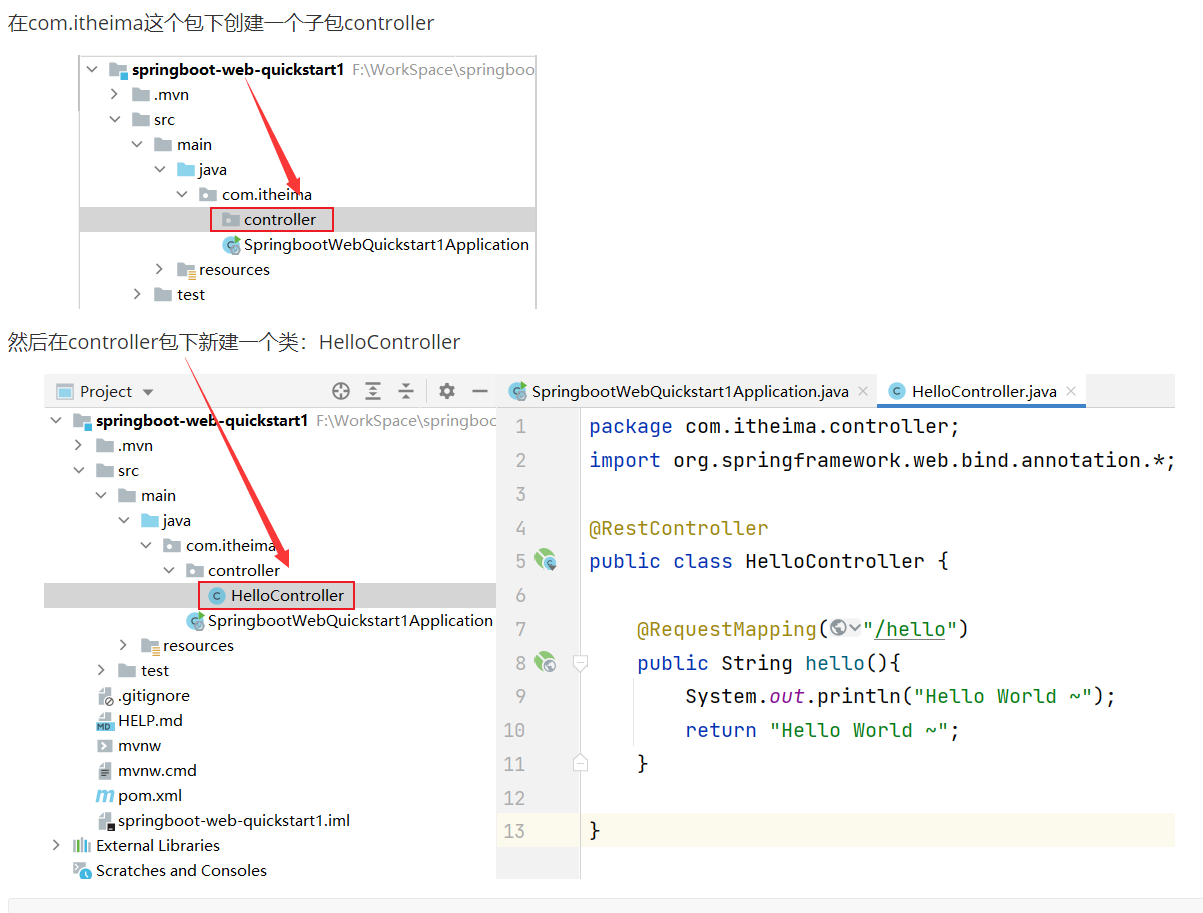
2.3 运行启动类
运行SpringBoot自动生成的引导类
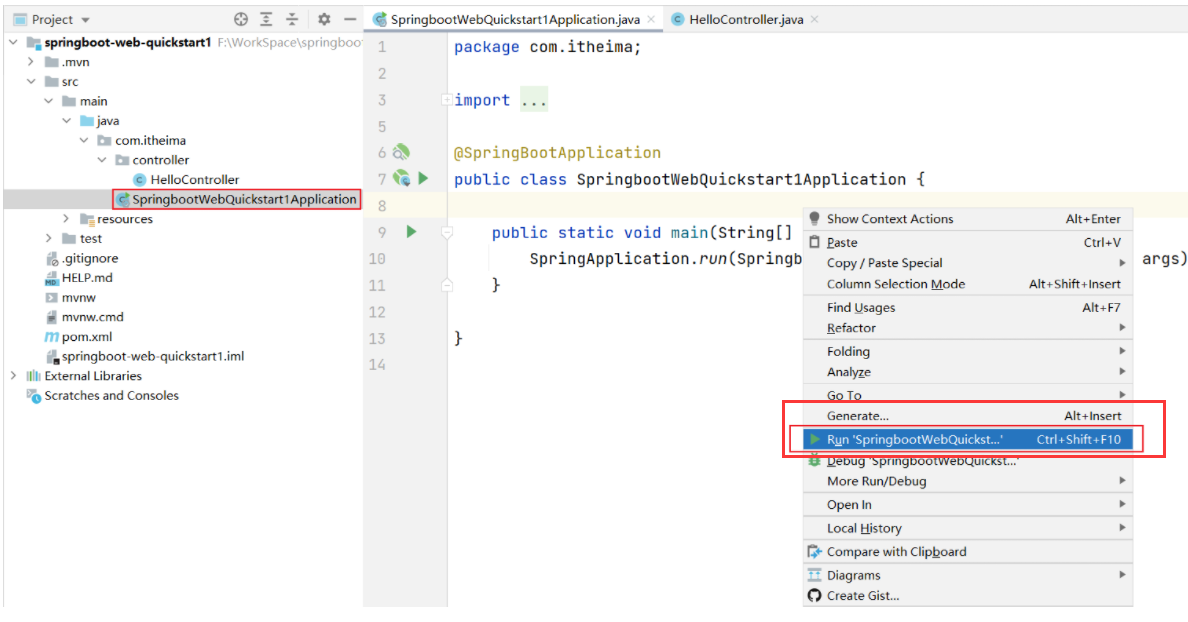
打开浏览器,输入 http://localhost:8080/hello
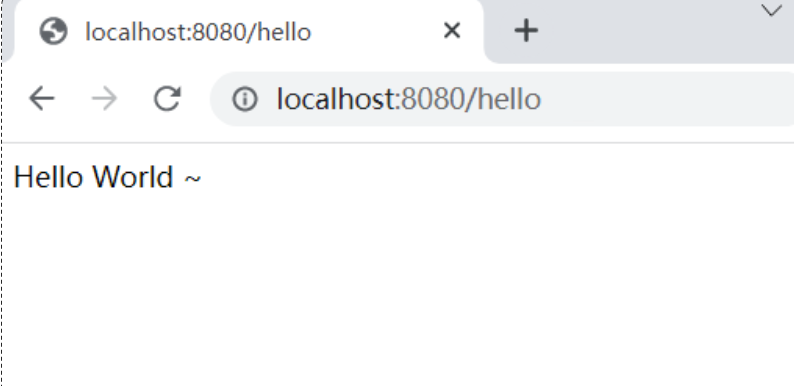
2.4 Web分析
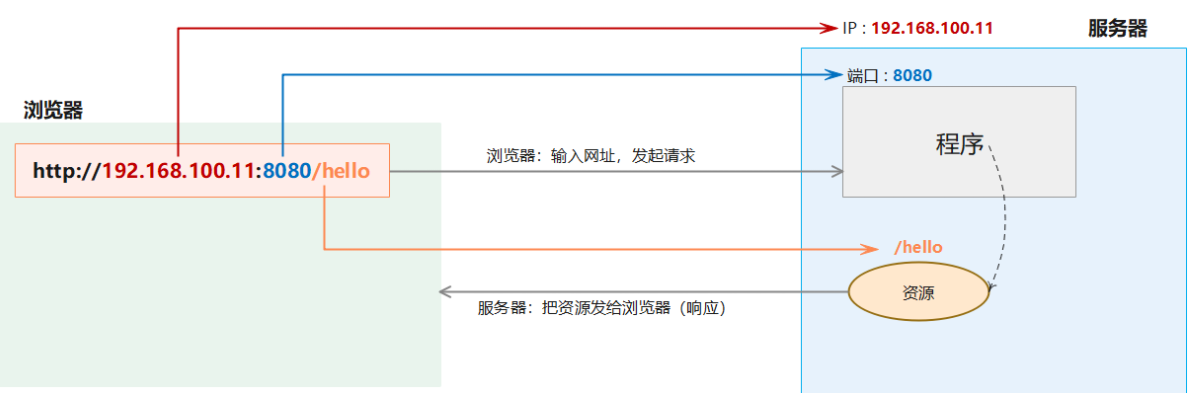
3. 配置文件(两种)
3.1 application.properties配置文件
3.2 application.yml配置文件
3.3 两者对比
3.4 配置文件书写和获取(yml为例)
1.第三方技术配置信息
2.自定义配置信息
书写:
获取:
1
2
3@Value("${键名}")
@ConfigurationProperties(prefix="共同上级/前缀")- 1:Value注解:
- 2.ConfigurationProperties注解(设定共同前缀):
4. 整合Mybatis
整体图
4.1 添加依赖
4.2 添加配置
4.3 书写业务逻辑
1.User类
- 实体类主要负责编写数据库的实体信息,和数据库的表要对应
1 | package com.itheima.springbootmybatis.pojo; |
2.UserMapper类
- 主要编写sql语句 ** **–主要用到Mapper和Select注解
1 | package com.itheima.springbootmybatis.mapper; |
3.UserService类
- 主要编写所有需求方法
1 | package com.itheima.springbootmybatis.Service; |
- 主要实现接口方法,调用Mapper类对象实现 –主要用到Service和Autowired注解
1 | package com.itheima.springbootmybatis.Service.impl; |
4.UserController类
- 主要调用Service接口对象实现 –主要用到RestController和Autowired和RequestMapping注解
1 | package com.itheima.springbootmybatis.controller; |
5.最终结果
5.Bean扫描(自动注解)
- Springboot启动类的注解可以自动扫描,并且默认扫描启动类所在包和子包
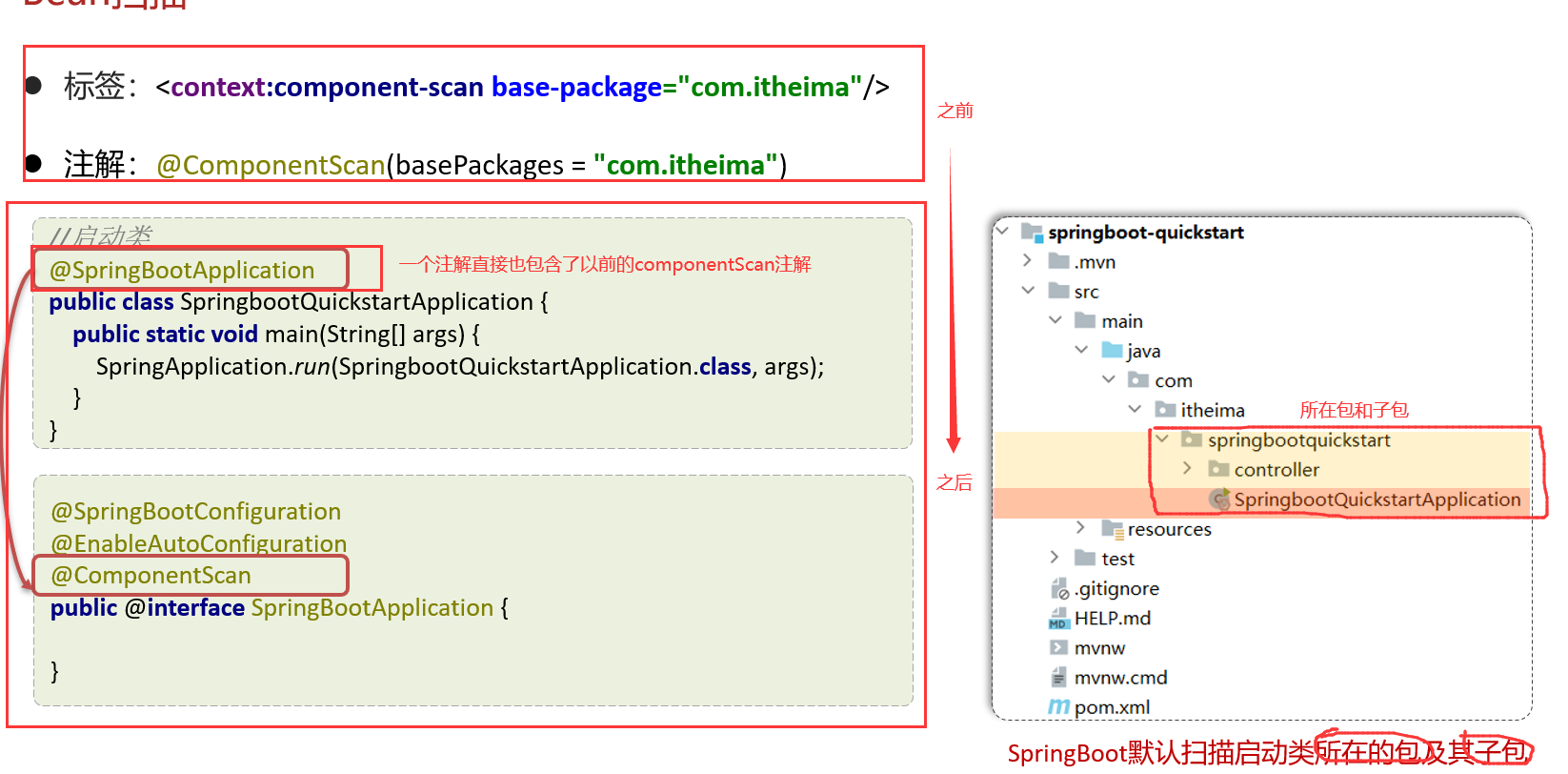
6.来自第三方的Bean对象
6.1 怎么引入
步骤一: maven下载
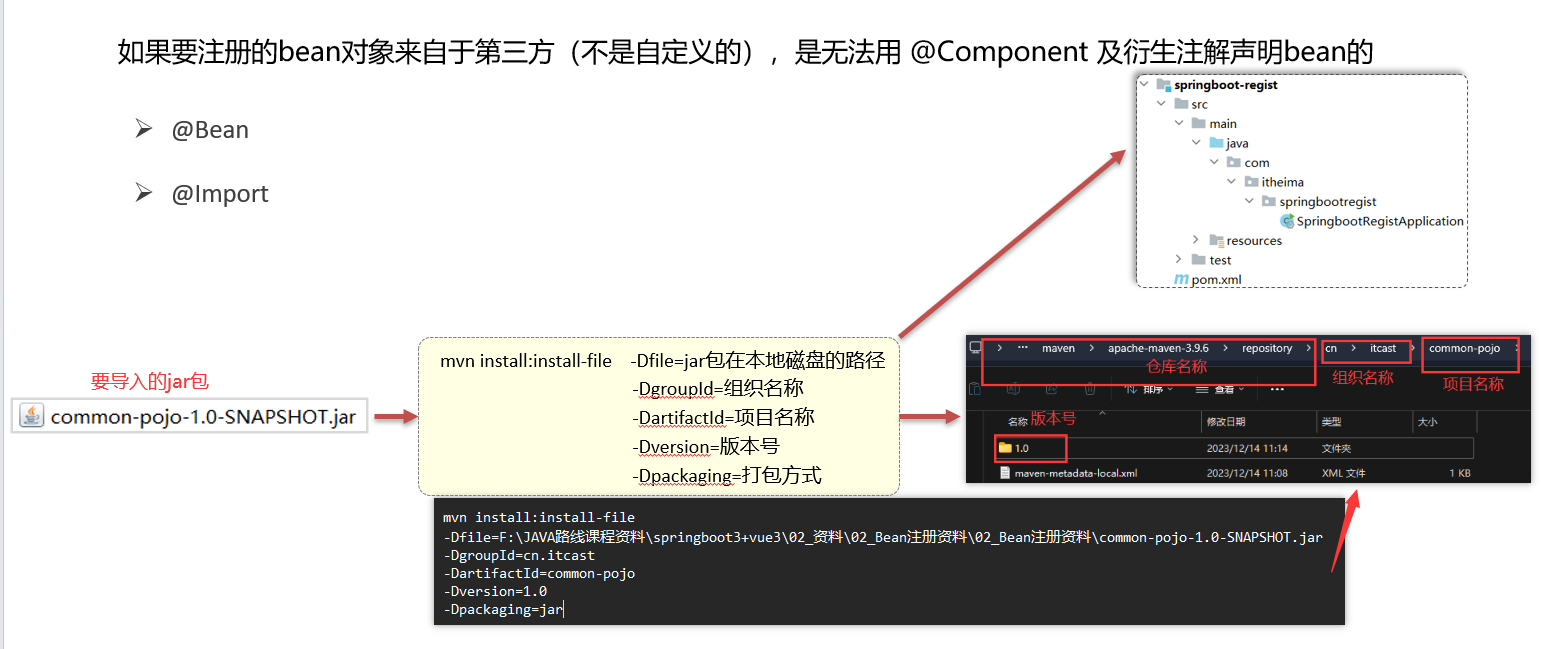
步骤二:pom.xml导入jar依赖
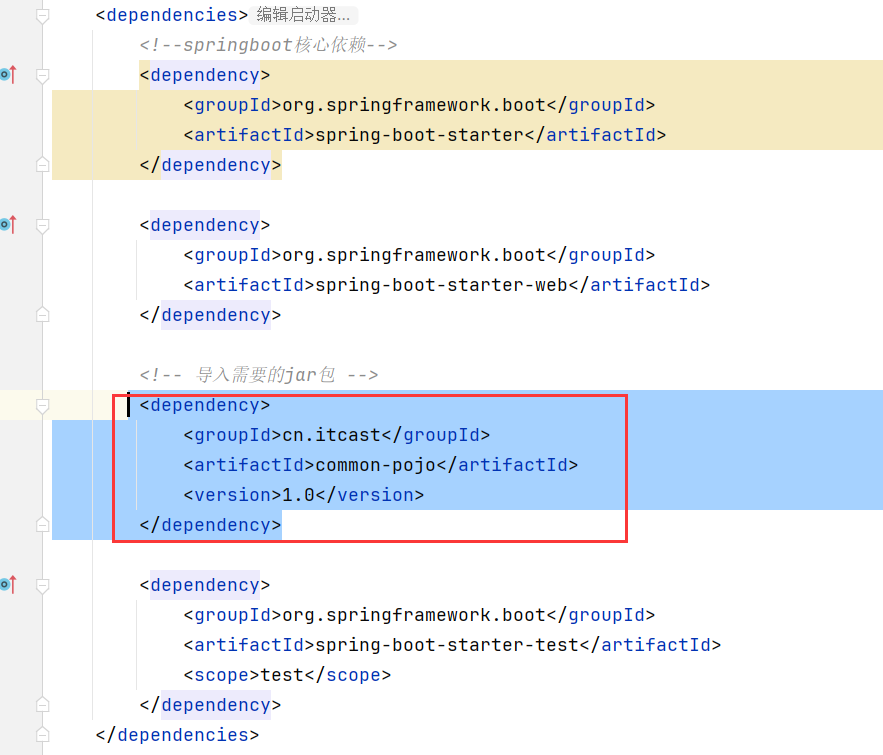
步骤三:刷新maven之后在外部库查看
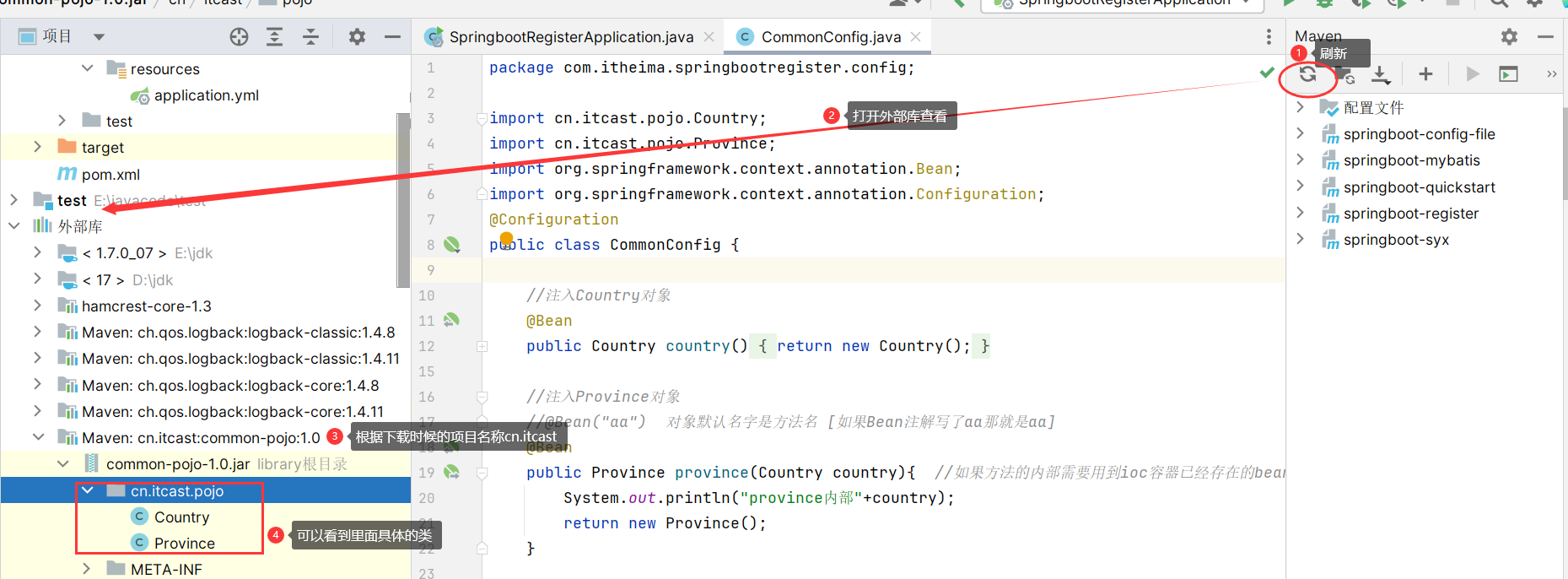
6.2 怎么注解
如果要注册的bean对象来自于第三方(不是自定义的),是无法用 @Component 及衍生注解声明bean的只能使用以下两种方式去解决bean注解
方式一:Bean注解
- 以下是两种方法的区别
- 1.存放到启动类
2.存放到配置类(@Configuration)
配置类是可以写很多bean对象,并且配置类必须存放在启动类的同级和下一级包位置,方便Bean扫描(如果放在外面就需要方法2import注解了)
方式二:Import注解
- 1.导入配置类
2.导入ImportSelector接口实现类
先创建一个java类重写ImportSelector接口的方法,返回值就是一个数组(要放入的bean对象)
然后在启动类中添加import注解就可以
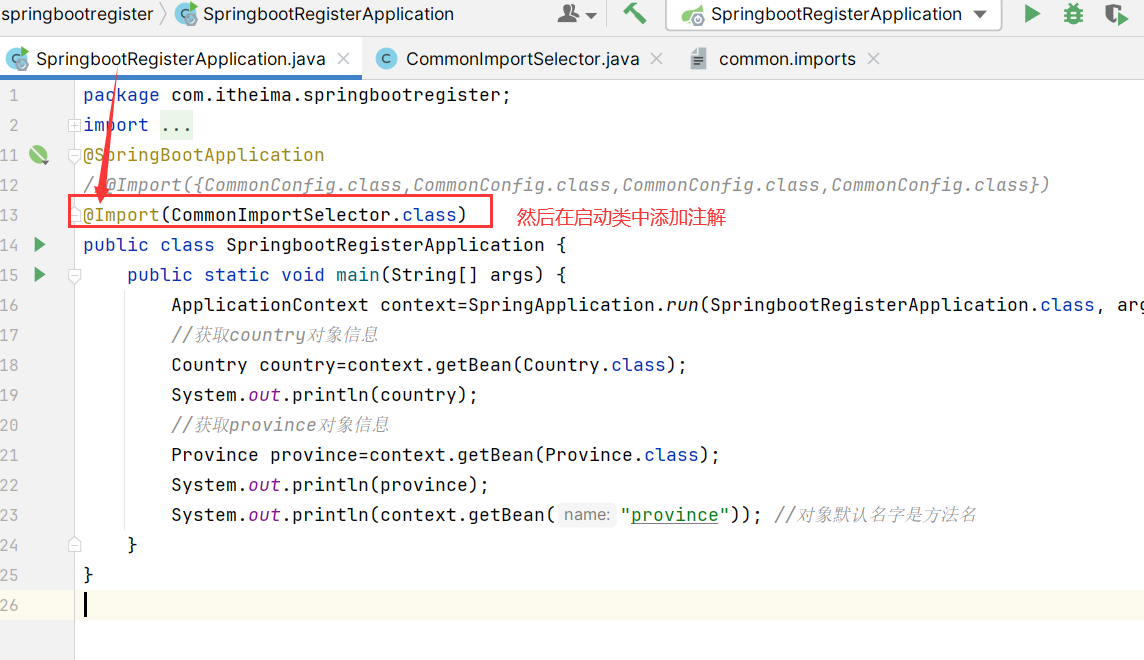
方式三: EnableXxxx注解(封装@Import注解)
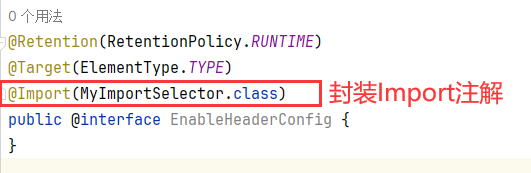
然后在启动类中添加import注解就可以
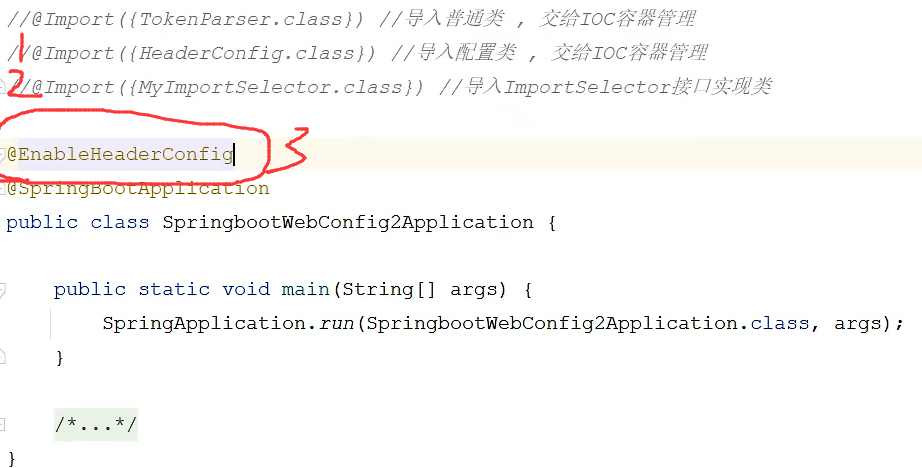
6.3 注册条件(符合条件才可以注入ioc容器)
Springboot提供了Conditional注解解决注册时候对象赋值问题,但是过于复杂所以给出了常用的三个相关注解
1. @ConditionalOnProperty(从配置文件判断)
从配置文件中的读取,读取成功注入,读取失败不注入
2.@ConditionalOnMissingBean(从IOC容器判断)
因为Country需要从配置文件中读取注入,失败了所以没有注入,这样的话下面的province就会注入
3.@ConditionalOnClass(从当前环境判断)
如果环境中有当前类就注入,没有就不注入
7.自动配置原理
7.1 原理图
- 其实就是将自动配置类写到一个.imports配置文件中
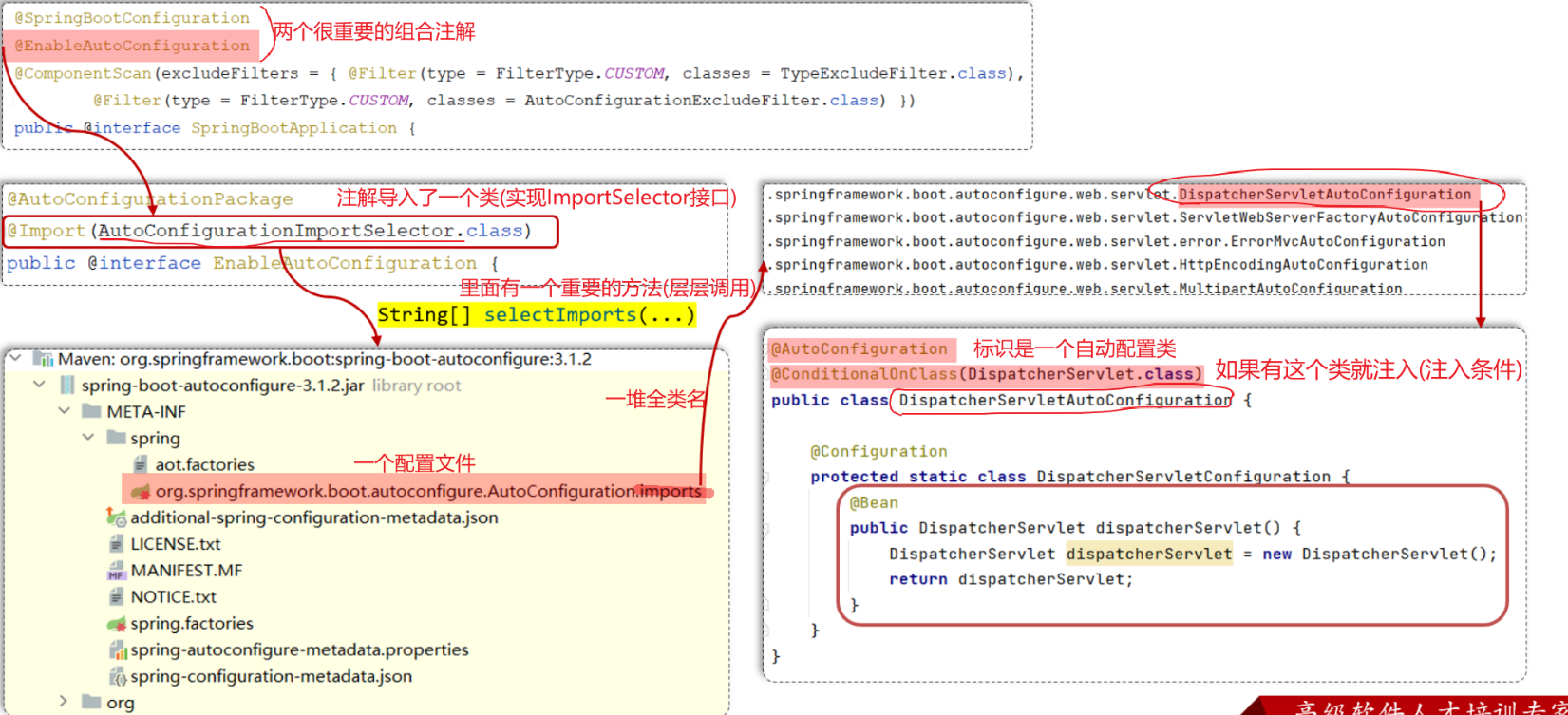
上图描述:
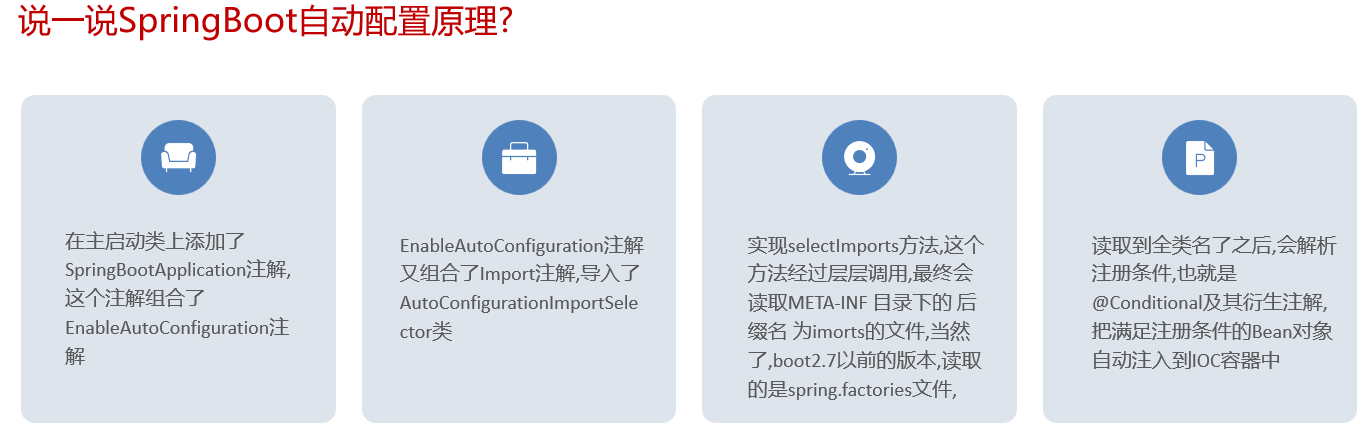
- 第二遍的理解
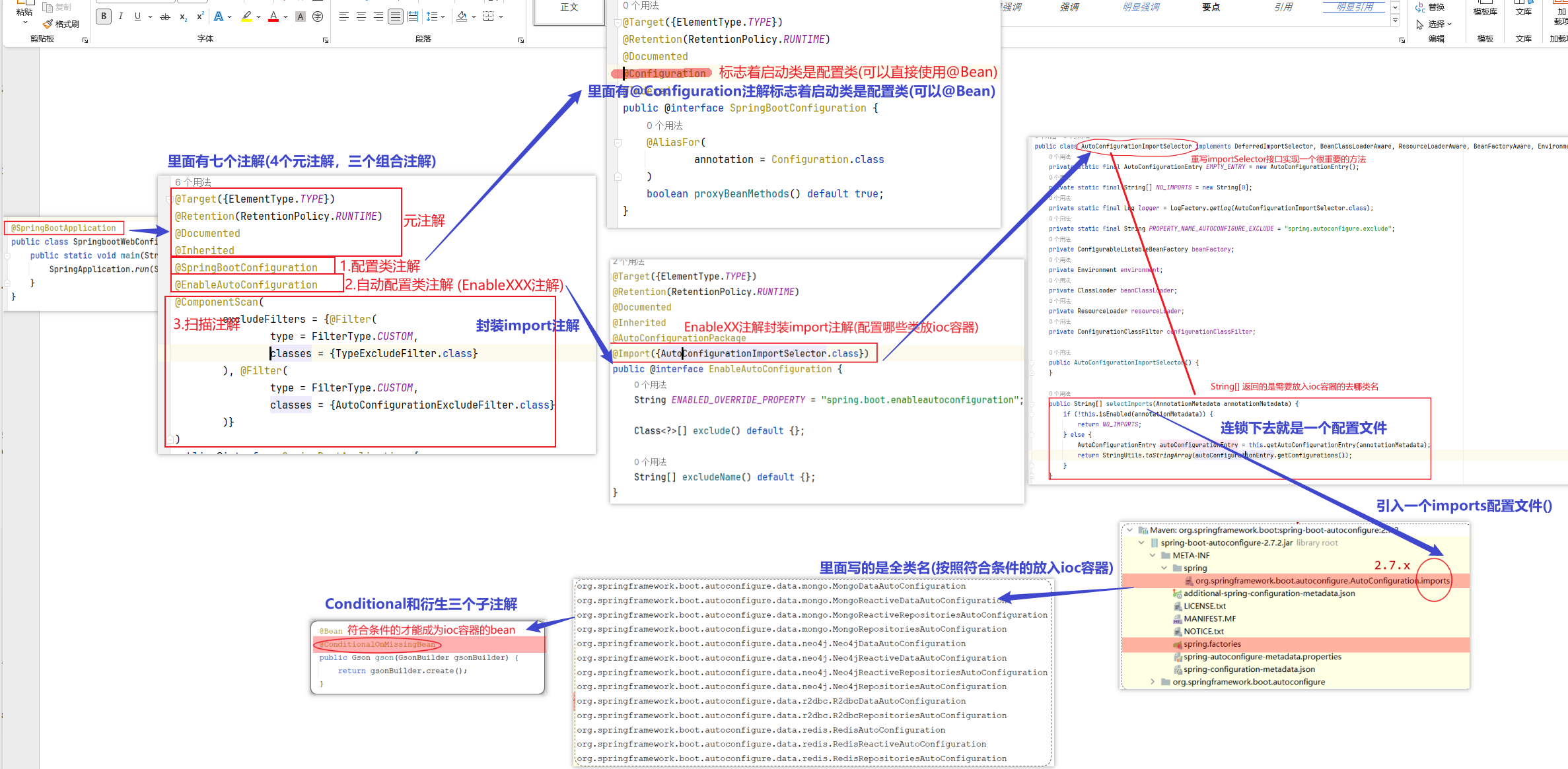
7.2 如何实现
- 原来的情况: 导入jar包之后只有类,需要自己写配置类和启动类import导入
现在的情况: 导入jar包的时候里面不仅仅有类,还有配置类,imports配置文件等,不需要添加任何其他操作
8.自定义starter(公共组件)
- 主要是自己写autoconfigure和starter两个组件
8.1 提供核心组件1-autoconfigure模块
8.2 提供核心组件2-starter模块
8.3 使用starter模块
9.Springboot父工程(为其他依赖提供兼容版本)
之前开发的SpringBoot入门案例中,我们通过maven引入的依赖,是没有指定具体的依赖版本号的。因为每一个SpringBoot工程,都有一个父工程。依赖的版本号,在父工程中统一管理
10.内置tomcat
我们的SpringBoot中,引入了web运行环境(也就是引入spring-boot-starter-web起步依赖),其内部已经集成了内置的Tomcat服务器。
我们可以通过IDEA开发工具右侧的maven面板中,就可以看到当前工程引入的依赖。其中已经将Tomcat的相关依赖传递下来了,也就是说在SpringBoot中可以直接使用Tomcat服务器
当我们运行SpringBoot的引导类时(运行main方法),就会看到命令行输出的日志,其中占用8080端口的就是Tomcat
11. Web后端开发总结
web后端开发现在基本上都是基于标准的三层架构进行开发的,在三层架构当中,Controller控制器层负责接收请求响应数据,Service业务层负责具体的业务逻辑处理,而Dao数据访问层也叫持久层,就是用来处理数据访问操作的,来完成数据库当中数据的增删改查操作。
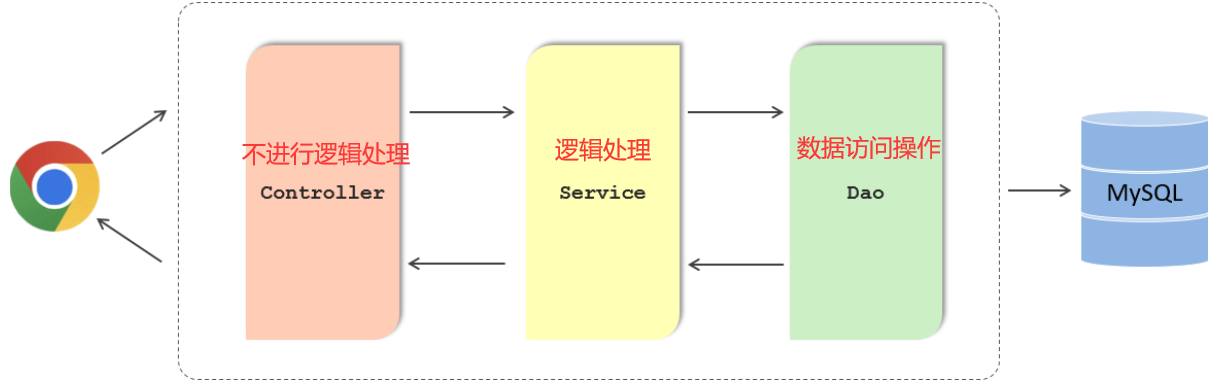
在三层架构当中,前端发起请求首先会到达Controller(不进行逻辑处理),然后Controller会直接调用Service 进行逻辑处理, Service再调用Dao完成数据访问操作
如果我们在执行具体的业务处理之前,需要去做一些通用的业务处理,比如:我们要进行统一的登录校验,我们要进行统一的字符编码等这些操作时,我们就可以借助于Javaweb当中三大组件之一的过滤器Filter或者是Spring当中提供的拦截器Interceptor来实现
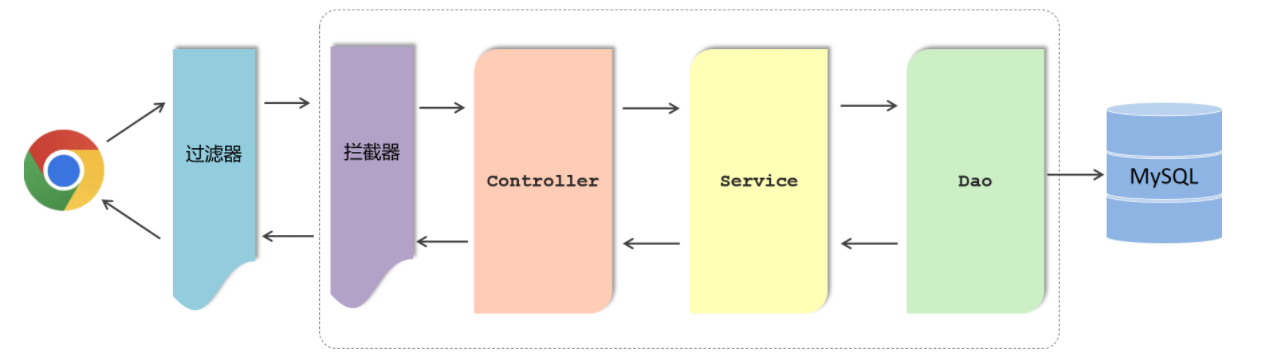
而为了实现三层架构层与层之间的解耦,我们学习了Spring框架当中的第一大核心:IOC控制反转与DI依赖注入
所谓控制反转,指的是将对象创建的控制权由应用程序自身交给外部容器,这个容器就是我们常说的IOC容器或Spring容器。
而DI依赖注入指的是容器为程序提供运行时所需要的资源
除了IOC与DI我们还讲到了AOP面向切面编程,还有Spring中的事务管理、全局异常处理器,以及传递会话技术Cookie、Session以及新的会话跟踪解决方案JWT令牌,阿里云OSS对象存储服务,以及通过Mybatis持久层架构操作数据库等技术
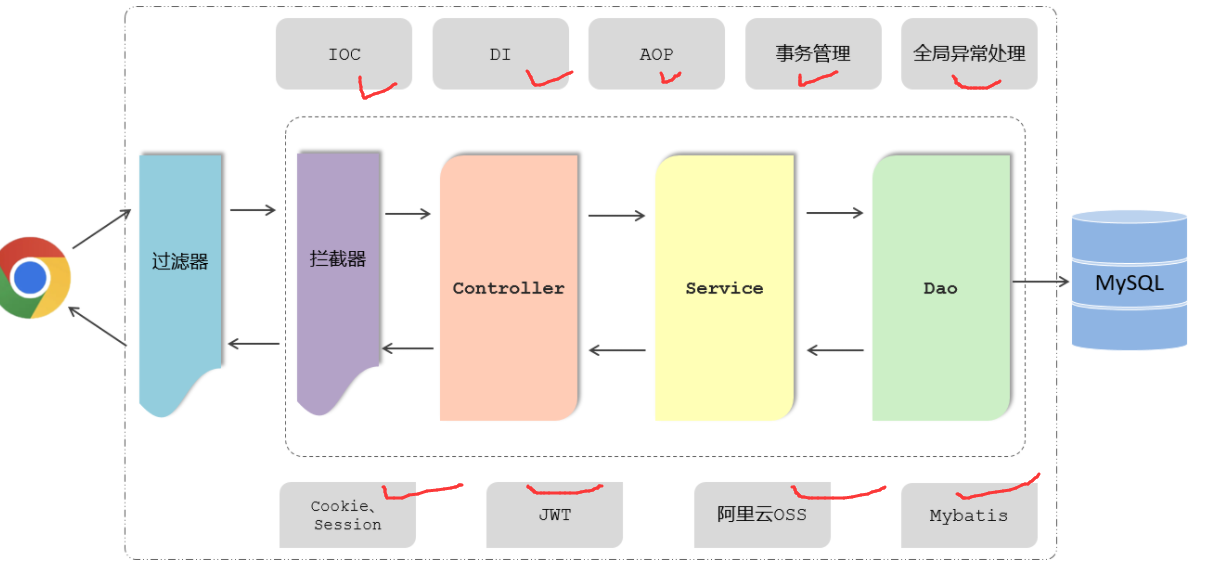
我们在学习这些web后端开发技术的时候,我们都是基于主流的SpringBoot进行整合使用的。而SpringBoot又是用来简化开发,提高开发效率的。像过滤器、拦截器、IOC、DI、AOP、事务管理等这些技术到底是哪个框架提供的核心功能
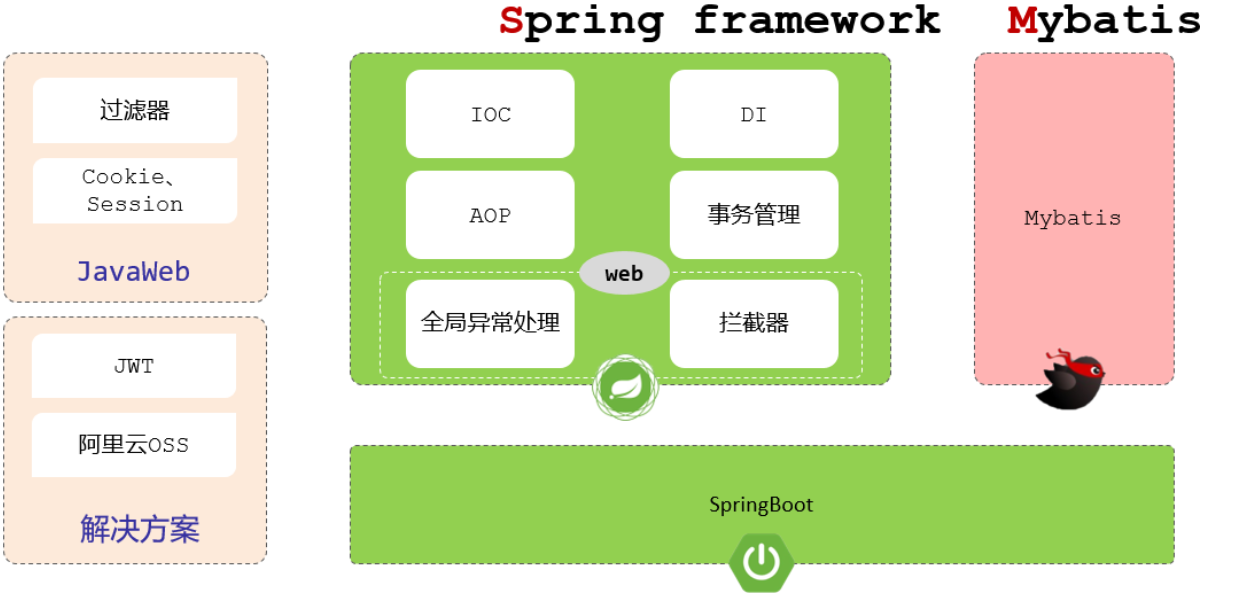
Filter过滤器、Cookie、 Session这些都是传统的JavaWeb提供的技术。
JWT令牌、阿里云OSS对象存储服务,是现在企业项目中常见的一些解决方案。
IOC控制反转、DI依赖注入、AOP面向切面编程、事务管理、全局异常处理、拦截器等,这些技术都是 Spring Framework框架当中提供的核心功能。
Mybatis就是一个持久层的框架,是用来操作数据库的。
在Spring框架的生态中,对web程序开发提供了很好的支持,如:全局异常处理器、拦截器这些都是Spring框架中web开发模块所提供的功能,而Spring框架的web开发模块,我们也称为:SpringMVC
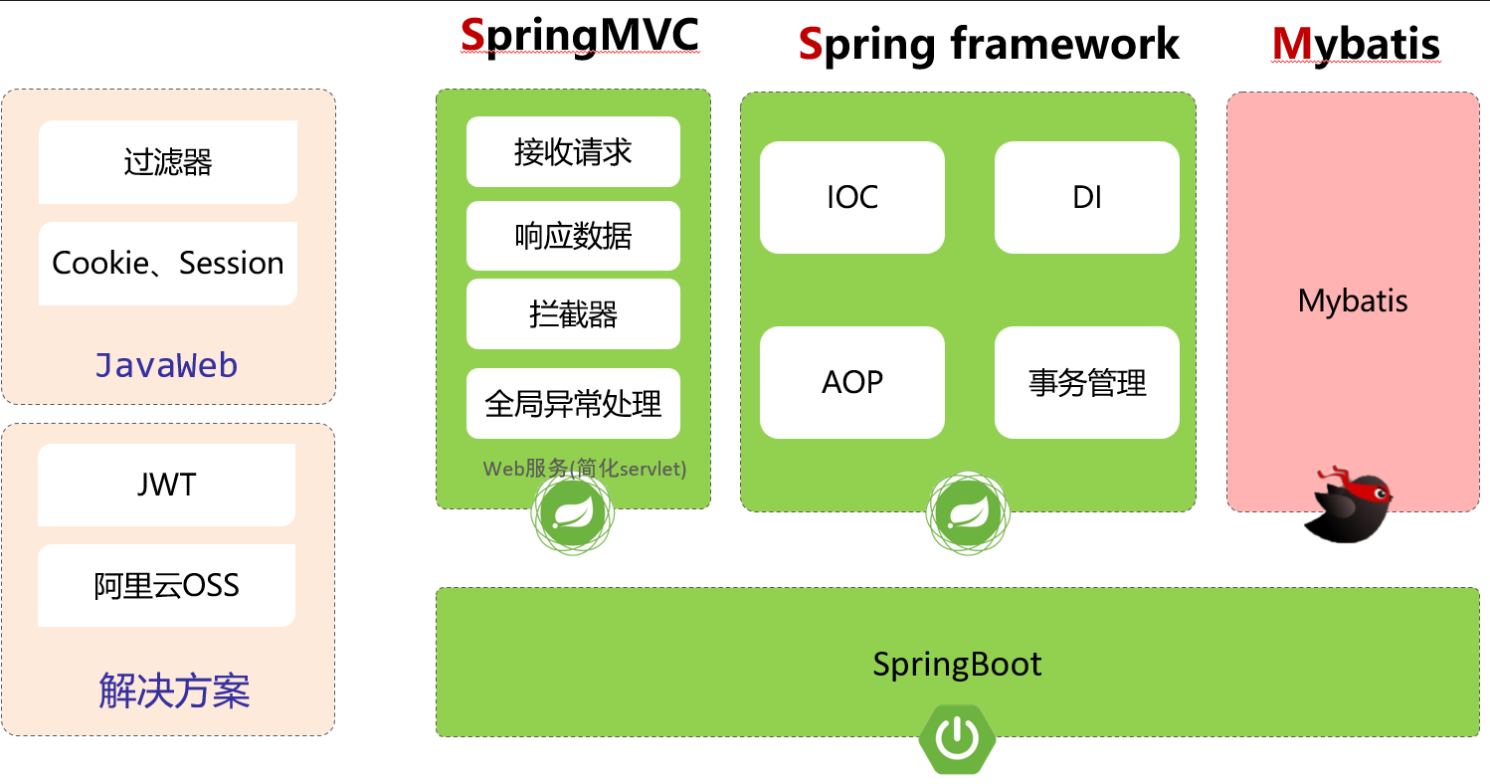
SpringMVC不是一个单独的框架,它是Spring框架的一部分,是Spring框架中的web开发模块,是用来简化原始的Servlet程序开发的
外界俗称的SSM,就是由:SpringMVC、Spring Framework、Mybatis三块组成。
基于传统的SSM框架进行整合开发项目会比较繁琐,而且效率也比较低,所以在现在的企业项目开发当中,基本上都是直接基于SpringBoot整合SSM进行项目开发的