删除排序链表的重复元素(改变指向)
题目
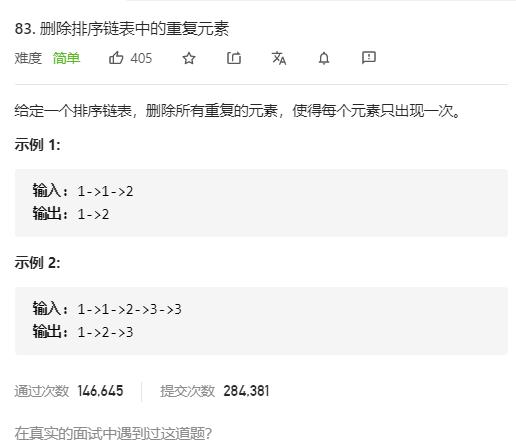
分析
1. 新建current链表指向head,方便后面移动
2. 只要当前节点值和下一个结点值相同就指向跳过下一个节点
3. 只要不相同就正常挪到下个节点判断
代码
1 2 3 4 5 6 7 8 9 10 11 12
| public static ListNode deleteDuplicates(ListNode head) { ListNode current = head; //要判断的链表给current while (current != null && current.next != null) { //没到结尾 if (current.next.val == current.val) { //当前节点和下一个结点值相同 current.next = current.next.next; //直接跳过下一个节点 } else { current = current.next; //不相同的话挪下一个结点判断 } } return head; //最后返回head即可 }
|
二进制链表转整数(倒序累积)
题目
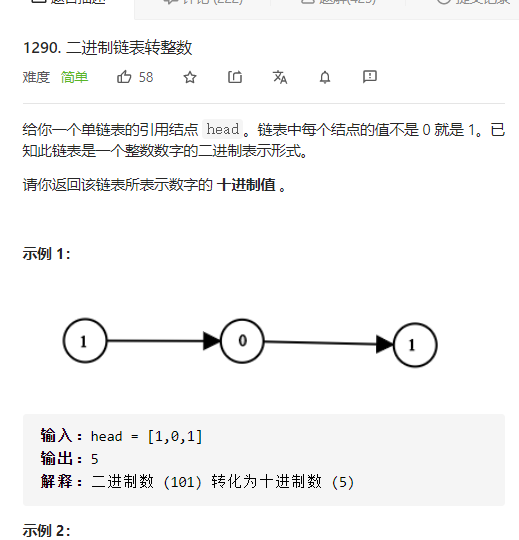
分析
1. 使用while循环测试出多少个节点(数组方便给大小)
2. 使用while循环将每一位给数组
3. 倒序将二进制累计!!
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| import java.util.*; public class Main { public static void main(String[] args) { Scanner input = new Scanner(System.in); ListNode head1=new ListNode(1); ListNode head2=new ListNode(0); ListNode head3=new ListNode(1); head1.next=head2; //生成三个节点 然后相连 head2.next=head3; System.out.println(getDecimalValue(head1)); //调用方法返回结果 } public static int getDecimalValue(ListNode head) { int res=0; int flag=0; //统计有几个节点 ListNode temp=head; while(temp!=null){ flag++; //+1 temp=temp.next; //节点往后遍历 }
temp=head; //重新指向head int[] a=new int[flag]; //存每一位 int t=0; //控制a数组下标 while(temp!=null){ a[t++]=temp.val; //记录当前值 temp=temp.next; //节点往后遍历 } System.out.println("a数组"+Arrays.toString(a));
int zs=0; //控制每一位数字乘2的倍数 for(int i=a.length-1;i>=0;i--){ System.out.println("a[i]是:"+a[i]+" 当前倍数乘的是:"+Math.pow(2,zs)); res+=a[i]*Math.pow(2,zs); zs++; } return res; }
}
|
结果
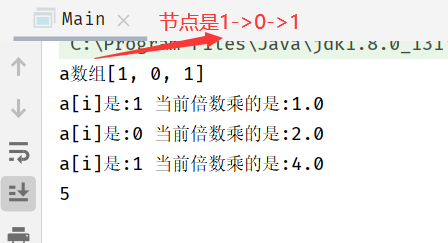