文件下载
1. 响应头没有设置:Content-Disposition(浏览器默认按照inline值处理)
1.1 inline 就是能显示就显示,不能显示就下载
2. 响应头设置:Content-Disposition="attachment;filename=文件名"
2.1 attachment 以附加形式下载
2.2 filename 就是下载时显示的下载文件名
导入jar包
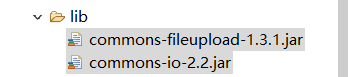
准备下载的文件
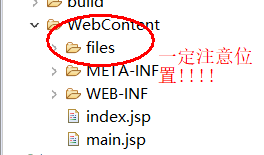
更改springmvc的静态资源
1 2 3
| //添加files文件的静态资源配置 <mvc:resources location="/files/" mapping="/files/**"> </mvc:resources>
|
index.jsp页面添加标签
1
| <a href="download?fileName=a.txt">下载</a>
|
注解类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| @Controller public class DemoController {
@RequestMapping("download") public void download(String fileName,HttpServletResponse res,HttpServletRequest req) throws IOException { //设置响应流中文件进行下载 res.setHeader("Content-Disposition","attachment;filename="+fileName); //把二进制流放入响应体中 ServletOutputStream os=res.getOutputStream(); //获取完整路径 String path=req.getServletContext().getRealPath("files"); //完整路径 //设置文件(路径,文件名) File file=new File(path,fileName); byte[] bytes=FileUtils.readFileToByteArray(file); //把一个文件导入转为二进制流 os.write(bytes); //流读入 os.flush(); os.close(); } }
|
执行项目点击下载
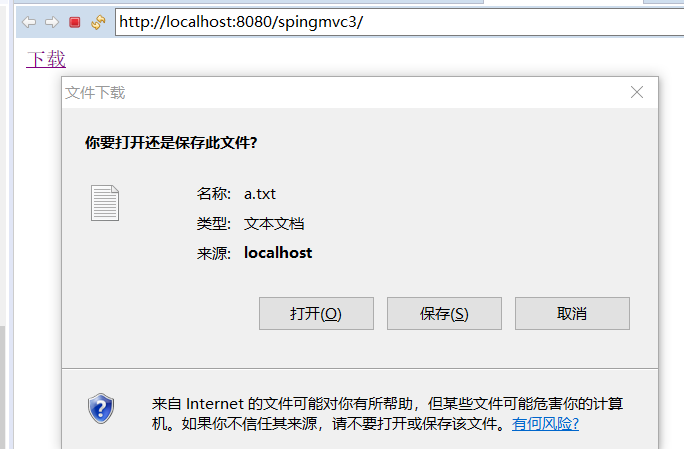
文件上传(MultipartResovler)
文件上传具体分析
1. 基于apache的commons-fileupload.jar完成文件上传
2. MultipartResovler作用
2.1 客户端上传的文件 -->MutipartFile封装类
2.2 通过MutipartFile封装类获取到文件流
表单数据类型(enctype属性)
//enctype属性取值:
1. application/x-www-form-urlencoded(默认值) :普通表单数据(少量文字信息)
2. text/plain :大量文字信息(邮件/论文)
3. multipart/form-data : 表单中包含二进制文件内容
导入jar包
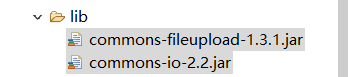
编写jsp页面
注解类传过去的类的对象和文件标签的name必须一致!!!
1 2 3 4 5
| <form action="upload" enctype="multipart/form-data" method="post"> //enctype属性取值是表单中包含二进制文件的值 姓名:<input type="text" name="name"/><br/> 文件:<input type="file" name="file"/><br/> //一定要和注解类对象名一致!!! <input type="submit" value="提交"/> </form>
|
编写springmvc.xml文件
编写MultipartResovler解析器和异常解析器
1 2 3 4 5 6 7 8 9 10 11 12 13
| <!-- MultipartResovler解析器 --> <bean id="multipartResovler" class="org.springframework.web.portlet.multipart.CommonsPortletMultipartResolver"> <property name="maxUploadSize" value="50"></property> //设置最大上传大小为50kb(超过我们就让弹出异常!!!) </bean> <!-- 异常解析器 --> <bean id="exceptionResolver" class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver"> <property name="exceptionMappings"> //使用exceptionMappings属性 <props> <prop key="org.springframework.web.multipart.MaxUploadSizeExceededException">/error.jsp</prop> //设置最大上传异常的提示给error.jsp文件 </props> </property> </bean>
|
注解类
注解类MultipartFile类的对象和文件标签的name必须一致!!!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| @Controller public class DemoController {
@RequestMapping() public String upload(MultipartFile file,String name) throws IOException { // MultipartFile类的文件file名必须和input里面的name="file"一致 String fileName = file.getOriginalFilename(); //获取文件名 String suffix = fileName.substring(fileName.lastIndexOf(".")); //使用substring获取到.后面文件 if(suffix.equalsIgnoreCase(".png")) { //不区分大小判断 String uuid = UUID.randomUUID().toString(); //获取随机后数字 FileUtils.copyInputStreamToFile(file.getInputStream(),new File("E:/"+uuid+suffix)); //上传到E盘 return "index.jsp"; } else{ return "error.jsp"; } } }
|