Spring整合Mybatis
导入jar包
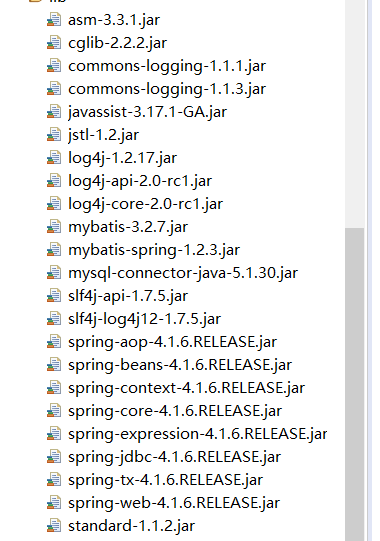
创建数据库
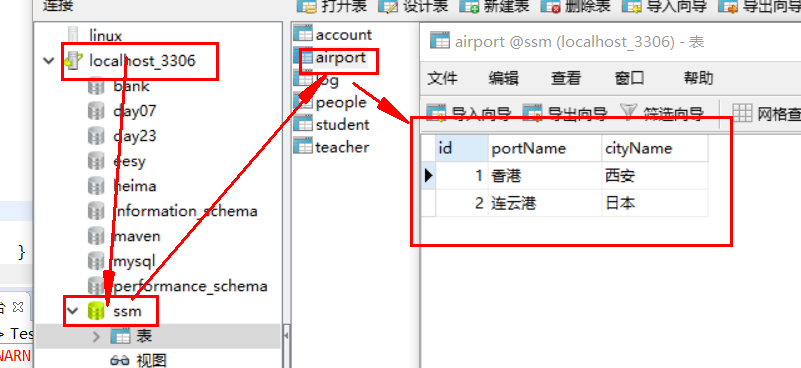
spring的bean标签代替mybatis全局配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| <?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- 数据封装类 替换mybatis里面连接数据库的文件 spring-jdbc.jar --> <bean id="dataSouce" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name="driverClassName" value="com.mysql.jdbc.Driver"></property> <property name="url" value="jdbc:mysql://localhost:3306/ssm"></property> <property name="username" value="root"></property> <property name="password" value="njdxrjgc7777777."></property> </bean> <!-- 创建SqlSessionFactory对象 --> <bean id="factory" class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="dataSource" ref="dataSouce"></property> <!-- 跳转到关于有数据库信息的bean --> </bean> <!-- 扫描器 相当于mybatis配置文件中mappers下的package标签 扫描mapper包下会给对应接口创建对象 --> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="basePackage" value="com.bjsxt.mapper"></property> <property name="sqlSessionFactory" ref="factory"></property> <!-- 跳转到factory的bean里面 --> </bean> </beans>
|
准备实体类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| public class Airport { private int id; private String portName; private String cityName; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getPortName() { return portName; } public void setPortName(String portName) { this.portName = portName; } public String getCityName() { return cityName; } public void setCityName(String cityName) { this.cityName = cityName; } @Override public String toString() { return "Airport [id=" + id + ", portName=" + portName + ", cityName=" + cityName + "]"; } }
|
使用接口绑定写sql语句
1 2 3 4 5 6
| public interface AirportMapper {
@Select("select * from airport") //注解的方式写sql语句 List<Airport> selAll(); //返回值是实体类的一个list形式 }
|
使用接口写查询结果方法
1 2 3
| public interface AirportService { List<Airport> show(); //返回值是实体类的一个list形式 }
|
使用实现类实现接口方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public class AirportServiceImpl implements AirportService { //添加接口实现 private AirportMapper airportMapper; //生成get和set方法 public AirportMapper getAirportMapper() { return airportMapper; }
public void setAirportMapper(AirportMapper airportMapper) { this.airportMapper = airportMapper; }
//实现service层接口方法 @Override public List<Airport> show() { return airportMapper.selAll(); } }
|
在配置文件中加bean
1 2 3 4 5
| <!-- 由spring管理service类 --> <bean id="airportService" class="com.bjsxt.service.impl.AirportServiceImpl"> <property name="airportMapper" ref="airportMapper"></property> <!-- 实现类里面的那个对象在这里配置 --> </bean>
|
test类
1 2 3 4 5 6 7 8 9 10 11 12 13
| public class Test { public static void main(String[] args) { //找spring容器配置文件地址 ApplicationContext ac=new ClassPathXmlApplicationContext("applicationContext.xml"); //默认从classes文件夹根目录开始寻找 //执行方法 AirportServiceImpl bean = ac.getBean("airportService",AirportServiceImpl.class); //通过bean去实例化 List<Airport> list = bean.show(); //调用接口实现类方法 System.out.println(list); } }
|
总结
代码框架
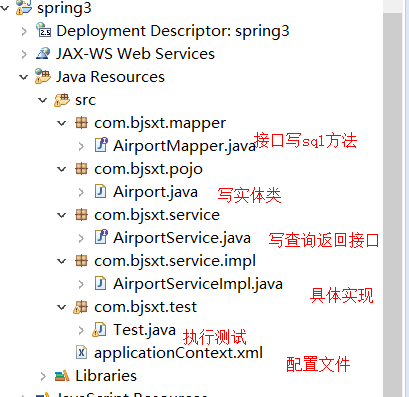
执行过程分析
1. 准备jar包和数据库
2. 准备好实体类(和数据库对的上)
3. 准备配置文件
1. 准备替换mybatis和数据库连接信息的bean
2. 准备刚才替换mybatis的文件的读入的bean
3. 准备创建扫描器(相当于mappers下面的package标签)
4. 准备要被spring管理的service类
4. 准备接口绑定的接口写sql语句
5. 准备service接口写返回的方法
6. 准备实体类实现接口返回结果
1. 要准备接口绑定接口的对象
2. 生成get和set方法
3. 实现接口方法 返回结果!!
7. 准备service的bean
8. 准备实现test测试类
1. 通过ApplicationContext找配置文件
2. 通过对象调用bean方法查找到配置文件中的关于service类的bean去找实现类实现
执行结果:
