自动登录分析
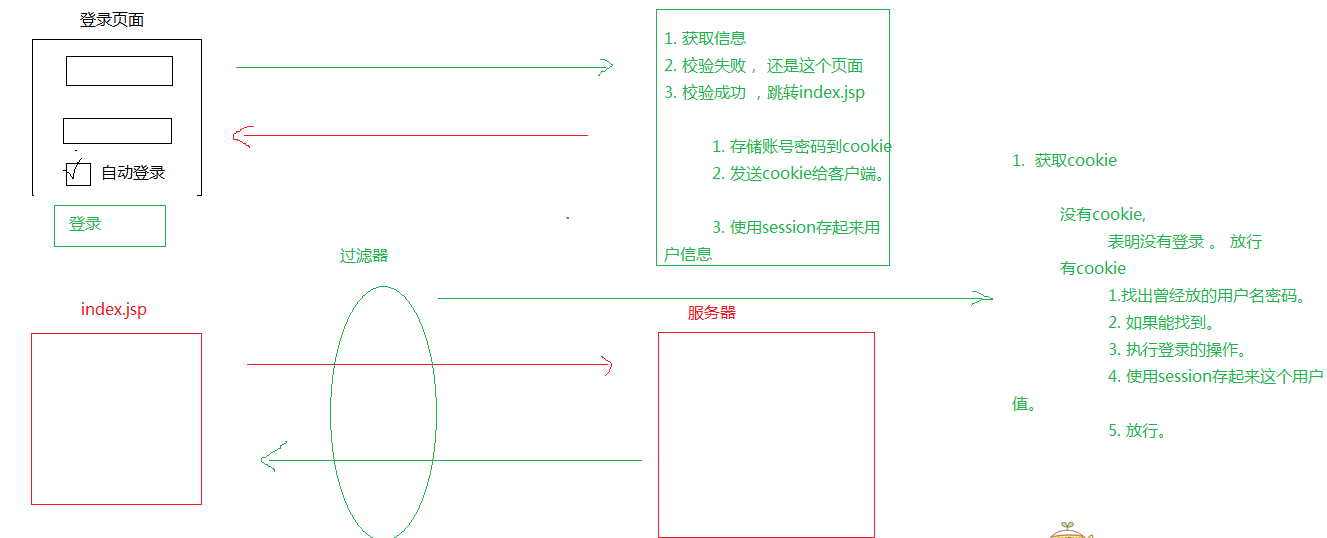
环境搭建
建立数据库
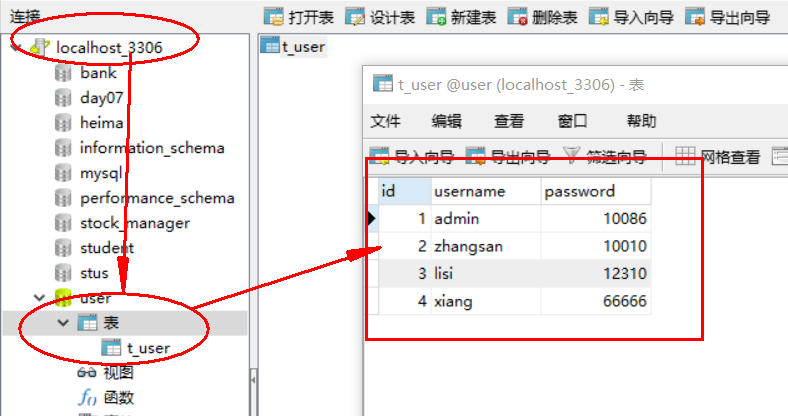
建立页面
1 2 3 4 5 6 7 8
| <body> <form method="post" action=""> 账号:<input type="text" name="username"><br> 密码:<input type="password" name="password"><br> <input type="checkbox" name="auto_login">自动登录<br> <input type="submit" value="登录"> </form> </body>
|
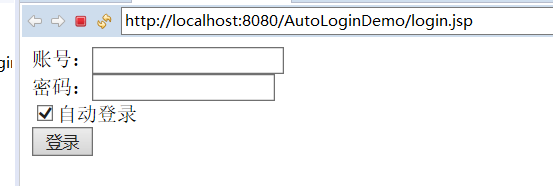
拷贝jar和JDBCUtils类,C3P0配置文件
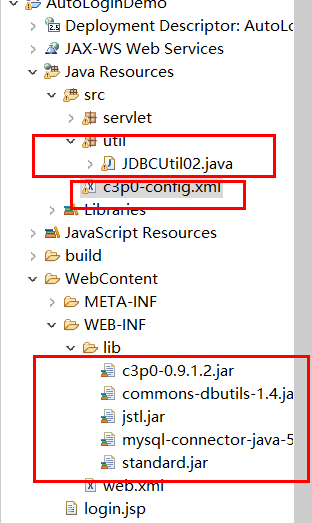
MVC模型各层实现
dao层代码(接口写方法)
1 2 3
| public interface UserDao { UserBean login(UserBean user) throws SQLException; //将传入的数据封装成一个对象 返回所有信息 }
|
UserBean层(数据封装)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| public class UserBean {
private int id; //数据库的所有属性 private String username; private String password; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
|
UserDaoImpl层(具体实现)
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public class UserDaoImpl implements UserDao { @Override public UserBean login(UserBean user) throws SQLException { //1.调用DBUtils的类 QueryRunner runner=new QueryRunner(JDBCUtil02.getDataSource()); //2.写sql语句 String sql="select * from t_user where username=? and password=?"; //3.调用query方法 return runner.query(sql,new BeanHandler<UserBean>(UserBean.class),user.getUsername(),user.getPassword()); } }
|
Servlet层
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| //1.获取数据 String username=request.getParameter("username"); String password=request.getParameter("password"); String autologin=request.getParameter("auto_login"); //测试控制台输出一下输入情况: System.out.println(username+"="+password+"="+autologin); UserBean user=new UserBean(); //将登陆界面的账号密码封装一下 user.setUsername(username); user.setPassword(password); //2.dao层实现 UserDao dao=new UserDaoImpl(); UserBean userBean=dao.login(user); //UserDao存的是 //3.判断跳转 if(userBean!=null) //成功进入首页 { request.getSession().setAttribute("userBean", userBean); response.sendRedirect("index.jsp"); //使用重定位 } else //输入不正确就还是这个界面 { request.getRequestDispatcher("login.jsp").forward(request, response); //使用转发
|
BeanUtils介绍
使用框架:
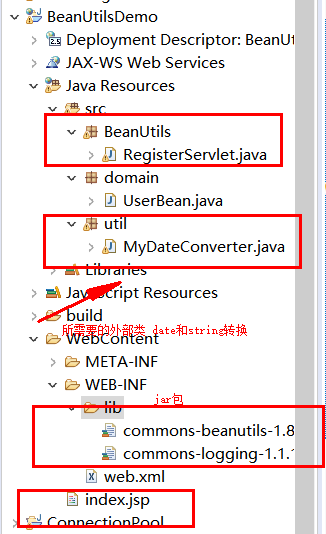
主页的jsp页面
1 2 3 4 5 6 7 8 9 10 11 12
| <body> <form method="post" action="RegisterServlet"> 账号:<input type="text" name="username"><br> 密码:<input type="password" name="password"><br> 邮箱:<input type="text" name="email"><br> 电话:<input type="text" name="phone"><br> 地址:<input type="text" name="address"><br> 生日:<input type="text" name="birthday"><br>
<input type="submit" value="注册"> </form> </body>
|
Servlet代码
1 2 3 4 5 6 7 8 9
| //注册自己的日期转换器 ConvertUtils.register(new MyDateConverter(), Date.class); //转化数据 Map map = request.getParameterMap(); UserBean bean = new UserBean(); BeanUtils.populate(bean, map);
System.out.println("22222bean="+bean.toString());
|
MyDateConverter类(具体用来String转Date)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public class MyDateConverter implements Converter {
@Override // 将value 转换 c 对应类型 // 存在Class参数目的编写通用转换器,如果转换目标类型是确定的,可以不使用c 参数 public Object convert(Class c, Object value) { String strVal = (String) value; // 将String转换为Date --- 需要使用日期格式化 DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd"); try { Date date = dateFormat.parse(strVal); return date; } catch (ParseException e) { e.printStackTrace(); } return null; } }
|

过滤器
思路:
过滤器的核心 帮用户完成登录的功能。
先判断session是否有效, 如果有效,就不用取cookie了,直接放行。
如果session失效了,那么就取 cookie。
没有cookie 第一次来 放行
有cookie
1. 取出来cookie的值,然后完成登录
2. 把这个用户的值存储到session中
3. 放行。
具体实现:
1. new一个新的Filter的文件,然后需要配置web里面的路径
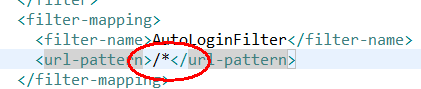
2. 编写取出cookie比对的工作类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class CookieUtil {
public static Cookie findCookie(Cookie[] cookies,String name) { //传入已经有的cookie和传进来的name比对 if(cookies!=null) { for(Cookie cookie:cookies) //循环 { if(name.equals(cookie.getName())) { return cookie; //匹配传过来的和存的有相同的 } } } return null; } }
|
3.编写思路实现(只实现doFilter()方法):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| public class AutoLoginFilter implements Filter { public AutoLoginFilter() {}
public void destroy() {}
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { try { HttpServletRequest r=(HttpServletRequest)request; //强转一下 //先判断,session中还有没有那个userBean UserBean userBean=(UserBean) r.getSession().getAttribute("userBean"); if(userBean !=null) //还有效 { chain.doFilter(request, response); //放行 } else //会话失效 { //1.从请求中取出cookie 但是cookie有很多key-value Cookie[] cookies=r.getCookies(); //2.从一堆的cookie里面找到之前的那个cookie Cookie cookie=CookieUtil.findCookie(cookies,"auto_login"); //servlet里面new的时候给的是 auto_login if(cookie==null) //第一次登陆 { chain.doFilter(request, response); //放行 } else //不是第一次 { //来到这里 表明什么情况 String value = cookie.getValue(); String username = value.split("#itheima#")[0]; String password = value.split("#itheima#")[1]; //完成登录 UserBean user = new UserBean(); user.setUsername(username); user.setPassword(password);
UserDao dao = new UserDaoImpl(); userBean = dao.login(user); //使用session存这个值到域中,方便下一次未过期前还可以用。 r.getSession().setAttribute("userBean", userBean); chain.doFilter(request, response); //放行 } } } catch (SQLException e) { // TODO 自动生成的 catch 块 e.printStackTrace(); chain.doFilter(request, response); //异常也要放行 } } public void init(FilterConfig fConfig) throws ServletException {}
}
|
总结
展示代码层次
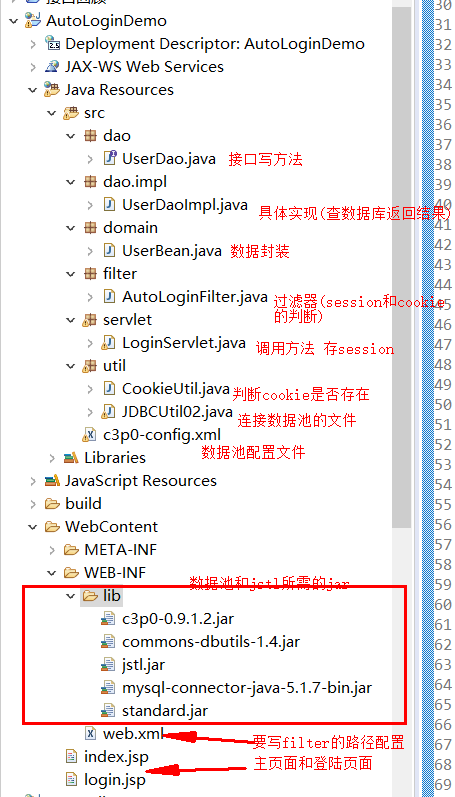
尝试使用:
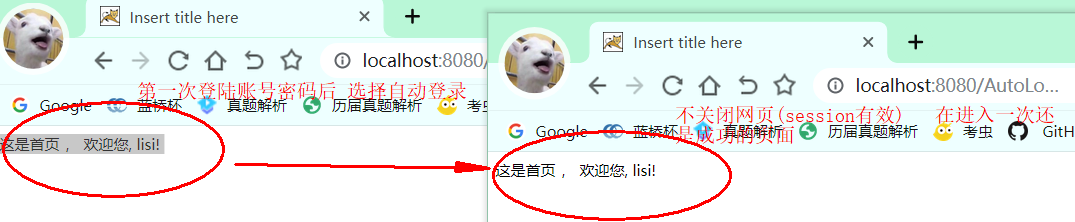