一、购物车实现分析
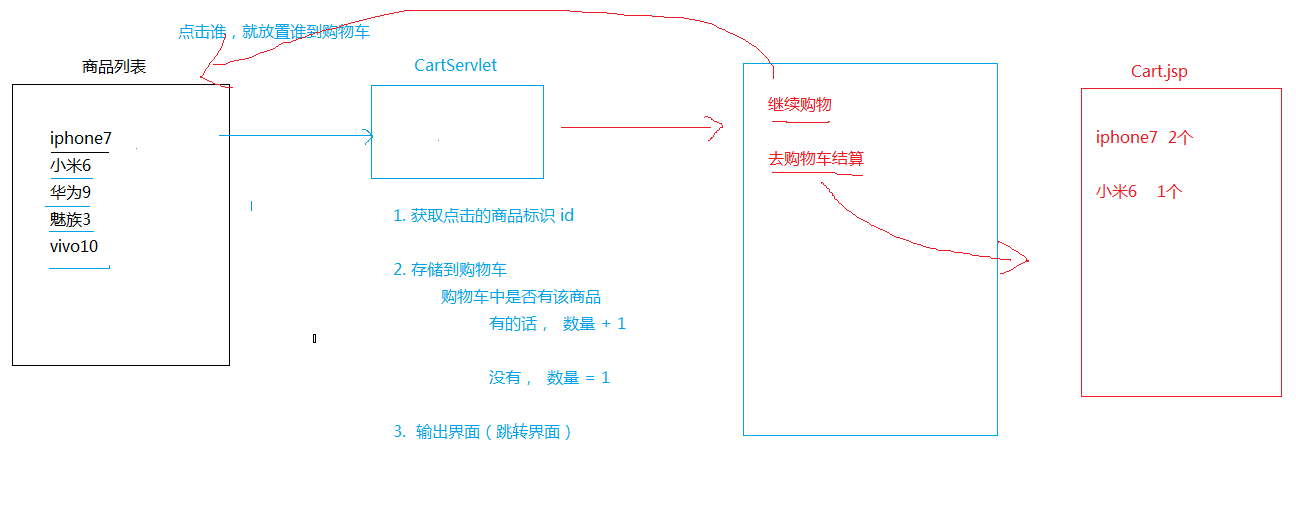
首先分为四个部分:分别是jsp的商品列表、CartServlet判断类,然后可以跳转商品列表的jsp界面和Cart.jsp界面(购物车结算)
CartServlet判断类:
1.先通过request.getParameter("id")获得jsp代码里面href里面的id号;然后通过names数组存放对应的手机名字
2.通过map集合存放对应的 手机号 数量
3.判断购物车有没有这个物品
4.跳转到对应的jsp页面去
二、购物车例子(Servlet实现)
jsp代码如下(使用三个标签跳转)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body>
<a href="CarServlet?id=0"><h3>Iphone 8</h3></a><br> <a href="CarServlet?id=1"><h3>小米6</h3></a><br> <a href="CarServlet?id=2"><h3>三星Note8</h3></a><br> <a href="CarServlet?id=3"><h3>魅族7</h3></a><br> <a href="CarServlet?id=4"><h3>华为9</h3></a><br> </body> </html>
|
CarServlet代码如下:
map在session里面:
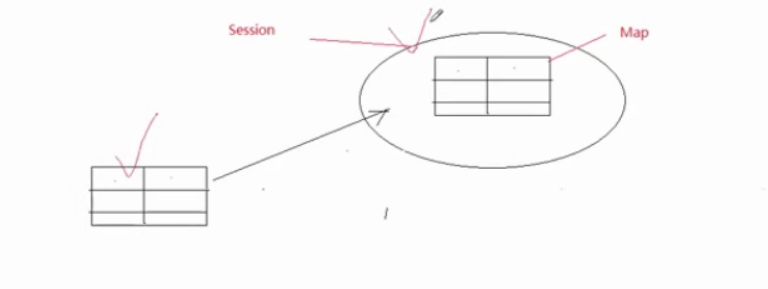
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| public class CarServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); //1.先获取要添加到购物车的商品id int id = Integer.parseInt(request.getParameter("id")); String[] names= {"Iphone 8","小米6","三星Note8","魅族7","华为9"}; //获取到id对应的商品名字 String name=names[id]; //2.获取购物车存放东西的session-----Map<String ,Integer> 比如Iphone7 3 Map<String,Integer> map=(Map<String, Integer>) request.getSession().getAttribute("cart"); //取值getAttribute if(map==null) { map=new LinkedHashMap<String,Integer>(); request.getSession().setAttribute("cart", map); //存值 } //3.判断购物车有没有这个物品 if(map.containsKey(name)) //map里面有一个遍历的方法 { //在原来基础上+1 map.put(name, map.get(name)+1); } else { //从来没买过直接设置为1 map.put(name, 1); } //4.输出界面(跳转) response.getWriter().write("<a href='product_list.jsp'><h3>继续购物</h3></a>"); response.getWriter().write("<a href='cart.jsp'><h3>去购物车结算</h3></a>"); }
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); }
}
|
点击对应的名字之后会弹出:
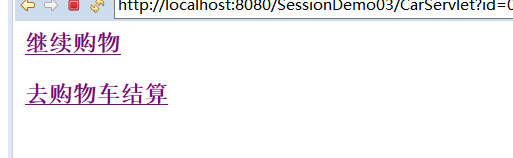
三、 展示购物车的东西:#
刚才就是点进去之后我们需要实现去购物车结算的功能,因此完成cart.jsp文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@page import="java.util.Map"%> <%@page import="java.util.Map"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body>
<h2>您的购物车的商品如下:</h2> <% //1.先获取map Map<String,Integer> map=(Map<String,Integer>)session.getAttribute("cart"); //2.遍历map if(map!=null) { for(String key:map.keySet()) //map.keySet()获取所有 { int value=map.get(key); //key:商品名称 //value:商品个数 //<h3>名称:Iphone 数量:6</h3> %> <h3>名称:<%=key %> 数量:<%=value %></h3> <br> <% } } %> </body> </html>
|
一开始我们设定一定量的苹果手机数量,然后点击小米-点击继续购物(添加到里面)-不停的重复-最终可以选择去购物车结算
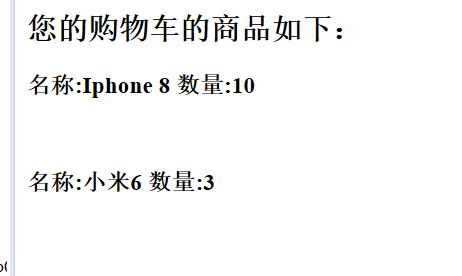
四、清空购物车#
- 在cart.jsp页面里面加一行清空购物车的按钮(按到展示页面之后可以选择清空购物车按钮,然后按钮可以跳转到clearCartServlet类,然后完成功能)
1
| <a href="clearCartServlet"><h4>清空购物车</h4></a>
|
- 而clearCartServlet类需要完成获取session然后移除掉之后可以重定定位跳转到product_list.jsp页面重新选择
清空session两种方式:
1 2 3 4 5 6
| //1.强制干掉会话,里面存放的任何数据就都没有了。 session.invalidate(); //2.从session中移除某一个数据 //session.removeAttribute("cart");
|
clearCartServlet类代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public class clearCartServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //获取session HttpSession session = request.getSession();
//移除值 session.removeAttribute("cart"); //使用重定位 response.sendRedirect("product_list.jsp"); //跳到product_list.jsp } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } }
|
五、完整分析和整理:
代码框架:
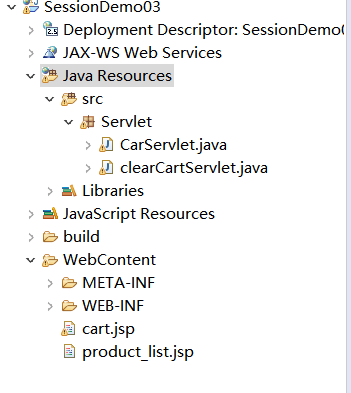
- product_list.jsp页面:主要是用来写首页的样子(手机名称)
- CarServlet类:主要是用来完成具体的操作;主要是完成点击商品之后获取商品id一存放商品,判断有没有然后增加可以跳转到继续购物(继续跳到首页)/购物车结算(cart.jsp页面)。
- cart.jsp页面:主要是完成跳转后看到购物车里面有哪些东西,然后可以选择清空购物车
- clearCartServlet类:主要是完成cart.jsp页面内的清空购物车,然后退出到首页。
六、具体代码如下
product_list.jsp页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body>
<a href="CarServlet?id=0"><h3>Iphone 8</h3></a><br> <a href="CarServlet?id=1"><h3>小米6</h3></a><br> <a href="CarServlet?id=2"><h3>三星Note8</h3></a><br> <a href="CarServlet?id=3"><h3>魅族7</h3></a><br> <a href="CarServlet?id=4"><h3>华为9</h3></a><br> </body> </html>
|
首页效果如下:
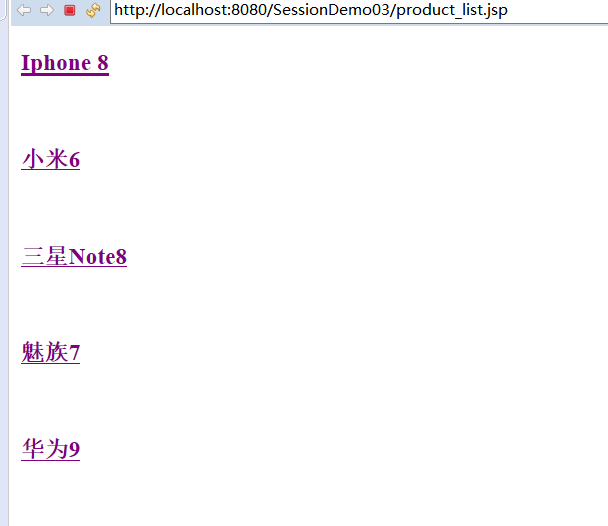
CarServlet类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| public class CarServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); //1.先获取要添加到购物车的商品id int id = Integer.parseInt(request.getParameter("id")); String[] names= {"Iphone 8","小米6","三星Note8","魅族7","华为9"}; //获取到id对应的商品名字 String name=names[id]; //2.获取购物车存放东西的session-----Map<String ,Integer> 比如Iphone7 3 Map<String,Integer> map=(Map<String, Integer>) request.getSession().getAttribute("cart"); //取值getAttribute if(map==null) { map=new LinkedHashMap<String,Integer>(); request.getSession().setAttribute("cart", map); //存值 } //3.判断购物车有没有这个物品 if(map.containsKey(name)) //map里面有一个遍历的方法 { //在原来基础上+1 map.put(name, map.get(name)+1); } else { //从来没买过直接设置为1 map.put(name, 1); } //4.输出界面(跳转) response.getWriter().write("<a href='product_list.jsp'><h3>继续购物</h3></a>"); response.getWriter().write("<a href='cart.jsp'><h3>去购物车结算</h3></a>"); }
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); }
}
|
商品点击后的二级页面如下:
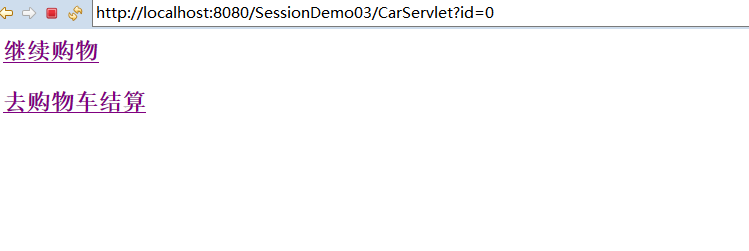
cart.jsp页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@page import="java.util.Map"%> <%@page import="java.util.Map"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body>
<h2>您的购物车的商品如下:</h2> <% //1.先获取map Map<String,Integer> map=(Map<String,Integer>)session.getAttribute("cart"); //2.遍历map if(map!=null) { for(String key:map.keySet()) { int value=map.get(key); //key:商品名称 //value:商品个数 //<h3>名称:Iphone 数量:6</h3> %> <h3>名称:<%=key %> 数量:<%=value %></h3> <br> <% } } %> <a href="clearCartServlet"><h4>清空购物车</h4></a> </body> </html>
|
商品点击清空购物车的页面如下:
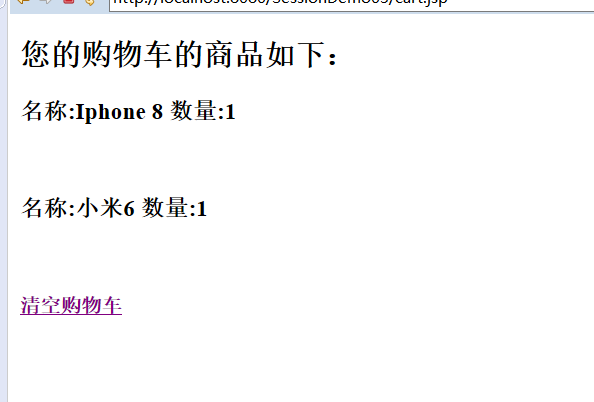
clearCartServlet类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public class clearCartServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //获取session HttpSession session = request.getSession(); //移除值 session.removeAttribute("cart"); //使用重定位 response.sendRedirect("product_list.jsp"); //跳到product_list.jsp } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } }
|