一、trigger和triggerHandler区别
trigger:触发事件,将光标移入框内(比较好)
triggerHandler:仅仅触发事件所对应的函数
完整代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> <script type="text/javascript" src="../js/jquery-1.11.0.js"></script> <script> $(function(){ $("#username").focus(function(){ console.log("text focus被触发了!"); //控制台输出一句话 }); $("#btn1").click(function(){ //第一个按钮的点击事件 //触发一下text的focus $("#username").trigger("focus"); }); $("#btn2").click(function(){ //第二个按钮的点击事件 //触发一下text的focus $("#username").triggerHandler("focus"); }); }); </script> </head> <body> 用户名:<input type="text" id="username"><br> <input type="button" value="triggerH触发一下focus" id="btn1"> <input type="button" value="triggerHandler触发一下focus" id="btn2"> </body> </html>
|
代码结果如下:
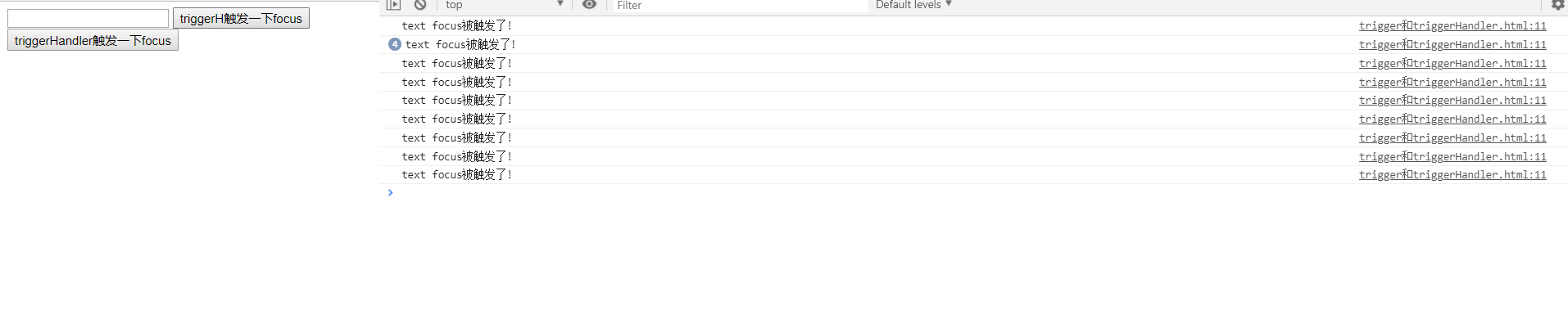
二、JQ方式实现表单检验
步骤分析:
1.导入相关JQ文件
2.文档加载事件:必填项后加一个小红点
3.表单校验确定事件: blur onfoucs onkeyup
4.提交表单:sumbit
具体操作分析:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| 1.进行导入相关JQ文件和css文件: <link rel="stylesheet" type="text/css" href="../css/style.css"/> <script type="text/javascript" src="../js/jquery-1.11.0.js" ></script>
2.放置好一个按钮和三个input然后去找css文件的high类给input的属性加红色*(利用after方法) $(".bitian").after("<font class='high'>*</font>");
3.给两个input设置blur事件(keyup.focus事件链式(triggerHandler()方法触发)) $(".bitian").blur(function(){}).focus(function(){}).keyup(function(){});
4.通过is()方法判断是密码还是用户名操作 &(this).is("#username"/"password")
5.判断之后提示使用append()方法输出(想要在*之后输出) $(this).parent().append("<span class='formtips onError'>密码太短了!</span>");
6.输出之后如果不清空(remove()方法)就一直弹出
$(this).parent().find(".formtips").remove();
7.最后就是整个表单提交(JS是所有的true才能提交),JQ是使用trigger("blur")去判断出错长度,出错少于0就成功 $(".bitian").trigger("blur"); //去blur测试会出现错误次数 var length=$(".onError").length;
|
具体代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79
| <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> <link rel="stylesheet" type="text/css" href="../css/style.css"/> <script type="text/javascript" src="../js/jquery-1.11.0.js" ></script> <script> $(function(){ //在后面加下红点* $(".bitian").after("<font class='high'>*</font>"); //调用css文件内的high类加载器变红色--在两个input后面加* //事件绑定 $(".bitian").blur(function(){ //取消焦点事件 var value=this.value; //清空当前必填项后面的span(不删除之前只要出错就弹出) $(this).parent().find(".formtips").remove(); //获得当前事件是谁触发 if($(this).is("#username")) { //校验用户名 if(value.length<6){ $(this).parent().append("<span class='formtips onError'>用户名太短了!</span>"); } else{ $(this).parent().append("<span class='formtips onSuccess'>用户名成功了!</span>"); } } if($(this).is("#password")) { //校验密码 if(value.length<3){ $(this).parent().append("<span class='formtips onError'>密码太短了!</span>"); } else{ $(this).parent().append("<span class='formtips onSuccess'>密码成功了!</span>"); } } }).focus(function(){ //链式 $(this).triggerHandler("blur"); }).keyup(function(){ $(this).triggerHandler("blur"); }); //给表单绑定提交事件 $("form").submit(function(){ //触发必选项的校验逻辑 $(".bitian").trigger("blur"); //去blur测试会出现错误次数 var length=$(".onError").length //去测试这个错误次数的个数 if(length>0){ return false; //如果有错误提示那么就不能提交 } return true; }); }); </script> </head> <body> <form action="../index.html" > //跳转到一个页面(自己选) <div> 用户名:<input type="text" class="bitian" id="username"> /放入同一类 </div> <div> 密码:<input type="password" class="bitian" id="password"> </div> <div> 手机号:<input type="tel"> </div> <div> <input type="submit"> </div> </form> </body> </html>
|
代码结果如下:
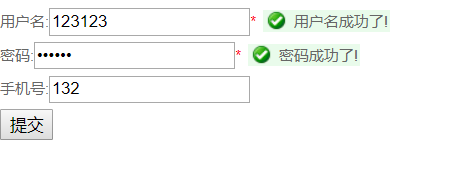