一、过滤器
选择标签第一个:##
主要代码分析:
1 2 3 4 5 6 7 8 9
| $(function(){ //2.文档加载事件(页面初始化操作) $(function(){ //3.给按钮绑定事件 $("#btn1").click(function(){ $("div:first").css("background-color","green"); //div标签里面的第一个改颜色 }); });
|
完整代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> <!--外部引入css文件--> <link rel="stylesheet" href="../../css/style.css"> <!--1.外部引入jq文件--> <script type="text/javascript" src="../../js/jquery-1.11.0.js" ></script> <script> //2.文档加载事件(页面初始化操作) $(function(){ //3.给按钮绑定事件 $("#btn1").click(function(){ $("div:first").css("background-color","green"); }); }); </script> </head> <body> <input type="button" value="过滤出所有div的第一个元素" id="btn1"><br /> <div id="one"> <div class="mini">1-1</div> </div> <div id="two"> <div class="mini">2-1</div> <div class="mini">2-2</div> </div> <div id="three"> <div class="mini">3-1</div> <div class="mini">3-2</div> <div class="mini">3-3</div> </div> <span id="four">span</span> </body> </html>
|
代码结果如下:
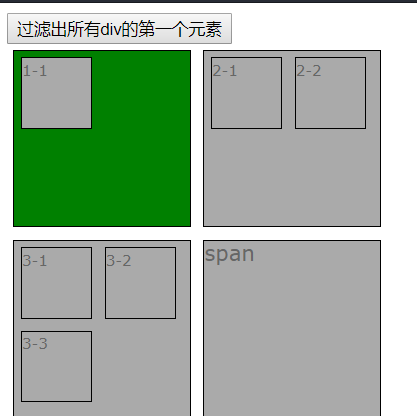
匹配所有索引值为偶数的元素(0开始):##
匹配所有索引值为奇数的元素(0开始):##
主要代码分析:
1 2 3 4 5 6 7 8 9 10 11
| $(function(){ //3.给按钮绑定事件 $("#btn1").click(function(){ $("div:even").css("background-color","green"); }); $("#btn2").click(function(){ $("div:odd").css("background-color","red"); }); });
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> <!--外部引入css文件--> <link rel="stylesheet" href="../../css/style.css"> <!--1.外部引入jq文件--> <script type="text/javascript" src="../../js/jquery-1.11.0.js" ></script> <script> //2.文档加载事件(页面初始化操作) $(function(){ //3.给按钮绑定事件 $("#btn1").click(function(){ $("div:even").css("background-color","green"); }); $("#btn2").click(function(){ $("div:odd").css("background-color","red"); }); }); </script> </head> <body> <input type="button" value="过滤出所有div中偶数位的div" id="btn1"><br /> <input type="button" value="过滤出所有div中奇数位的div" id="btn2"><br /> <div id="one"> <!--0--> <div class="mini">1-1</div> <!--1--> </div> <div id="two"> <!--2--> <div class="mini">2-1</div> <!--3--> <div class="mini">2-2</div> <!--4--> </div> <div id="three"> <!--5--> <div class="mini">3-1</div> <!--6--> <div class="mini">3-2</div> <!--7--> <div class="mini">3-3</div> <!--8--> </div> <span id="four">span</span> </body> </html>
|
代码结果如下:
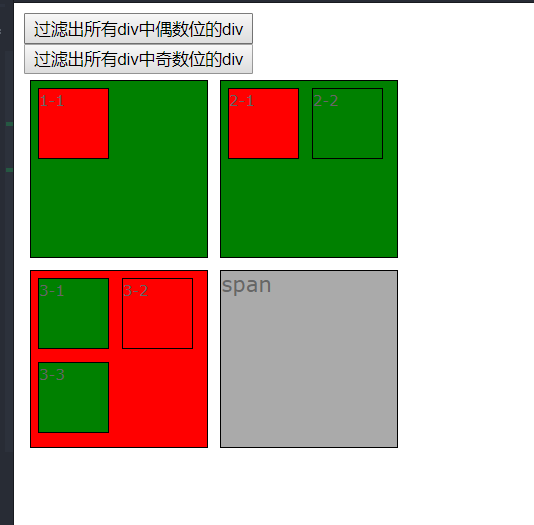
匹配所有大于(>)给定索引值(n)的元素:##
只展示部分代码(完整代码只需要把上面的div中value值和点击事件内的div:odd更改为div:gt(2))
1 2 3 4 5 6 7 8
| $(function(){ //3.给按钮绑定事件 $("#btn1").click(function(){ $("div:gt(2)").css("background-color","green"); }); });
|
代码结果如下:
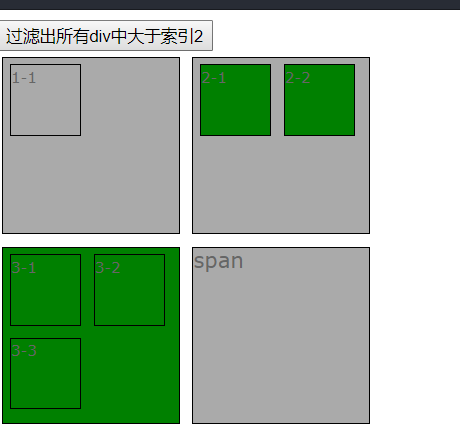
二、表单选择器
1
| $("input/text/textarea等表单元素")
|
主要代码如下:
1 2 3
| $(function(){ $("text").css("color","red"); });
|
完整代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> <!--1.外部引入jq文件--> <script type="text/javascript" src="../../js/jquery-1.11.0.js" ></script> <script> $(function(){ $("text").css("color","red"); }); </script> </head> <body> <form> <input type="button" value="Input Button"/> <input type="checkbox" /> <input type="file" /> <input type="hidden" /> <input type="image" /> <input type="password" /> <input type="radio" /> <input type="reset" /> <input type="submit" /> <input type="text" /> <select><option>1</option></select> <textarea></textarea> <button>Button</button> </form> </body> </html>
|
三、表单内涂色
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> <!--1.外部引入jq文件--> <script type="text/javascript" src="../../js/jquery-1.11.0.js" ></script> <script> $(function(){ $(":text").css("background-color","blue"); //text图背景色 $("#btn1").click(function(){ $("select option:selected").css("background-color","red"); //选中的项目选中后变红色 }); }); </script> </head> <body> <form> <input type="button" value="Input Bustton"/> <input type="checkbox" /> <input type="file" /> <input type="hidden" /> <input type="image" /> <input type="password" /> <input type="radio" /> <input type="reset" /> <input type="submit" /> <input type="text" /> <select multiple="multiple"> <!--多行显示--> <option>Option1</option> <option>Option2</option> <option>Option3</option> </select> <input type="button" value="点我" id="btn1" /> <textarea></textarea> <button>Button</button> </form> </body> </html>
|
代码结果如下:
